Top Tips for Maximizing React App Performance
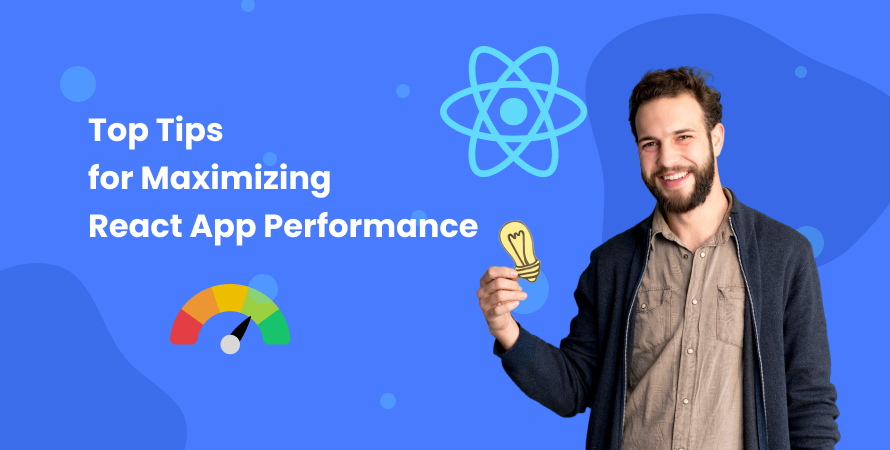
React app performance optimization techniques are crucial for delivering a smooth user experience. Faster load times and responsive interfaces not only enhance user satisfaction but also contribute significantly to higher SEO rankings. Improved performance can lead to reduced bounce rates, increased conversions, and even lower hosting costs.
In this article, we will explore top tips to maximize the performance of your React app by optimizing hook rendering. From understanding React’s rendering process to implementing advanced techniques like windowing and memoization, we’ll cover it all to ensure your app runs React faster and more efficiently than ever before.
Pre-Optimization Techniques and Performance Research
Before we get into specific performance optimization techniques, let’s talk about why pre-optimization is so important. Pre-optimization is all about identifying potential performance issues and understanding how your app currently performs. This process helps you figure out which areas need the most work and ensures that your optimization steps are effective.
Here are some key benefits of pre-optimization:
- Bottlenecks: By knowing where your app is slowing down, you can focus on those areas first.
- Setting Baselines: Establishing a performance baseline gives you a starting point to measure the impact of your optimizations.
- Resource Allocation: It helps you allocate your time and resources efficiently towards the most important optimizations.
Analyzing App Performance Using Lighthouse or WebPageTest
To start with pre-optimization, using tools like Lighthouse or WebPageTest can provide valuable insights into your app’s performance.
Lighthouse
Lighthouse is an open-source tool that helps you improve the quality of your web pages. It looks at things like performance, accessibility, and SEO. Here’s how you can use it:
- Open Chrome DevTools.
- Go to the “Lighthouse” tab.
- Click “Generate report.”
Lighthouse will analyze your app and give you a detailed report on what can be improved.
WebPageTest
WebPageTest is another powerful tool for measuring web performance. It has some advanced features like video capture and running tests from different locations around the world. Here’s how you can use it:
- Visit WebPageTest.
- Enter your app’s URL.
- Choose the test settings (like location and browser).
- Run the test to get a detailed report on load times, rendering issues, and suggestions for improvement.
Understanding React UI Updates, Virtual DOM, and Reconciliation
React’s approach to handling UI updates is designed for efficiency and performance. At its core is the Virtual DOM, an in-memory representation of the actual DOM. This abstraction layer allows React to manage changes more predictably and efficiently.
When a component’s state or props change, React optimization creates a new Virtual DOM tree. It doesn’t immediately update the actual DOM. Instead, it compares this new tree with the previous one using a process called diffing. Only after identifying what has changed does React update the real DOM, ensuring minimal and precise modifications.
The Reconciliation Process in React’s Rendering Algorithm
The reconciliation process is key to React’s performance optimization strategy. During reconciliation, React determines how to apply changes from the Virtual DOM to the real DOM with minimal overhead.
Here’s a breakdown of this React JS performance process:
- Diffing Algorithm: React compares the old Virtual DOM with the new one to identify changes.
- Batch Updates: Instead of updating the DOM for each change, React batches updates together, reducing reflows and repaints.
- Key Prop: In lists, using a stable key prop helps React identify which items have changed, been added, or removed, optimizing the React application.
Localizing Component State for Performance Optimization
To enhance the performance of your React app, it’s crucial to localize component states effectively. By keeping the state close to where it’s used, you can minimize unnecessary re-renders and ensure that your components remain efficient.
Memoizing Components
Memoization is a technique used to cache the results of expensive function calls and reuse them when the same inputs occur again. In React, this can be achieved using React.memo(), useCallback(), and useMemo().
React.memo()
This higher-order component optimizes functional components by memoizing their output. If the component’s props do not change, React skips rendering and reuses the last rendered result.
jsx const MyComponent = React.memo((props) => { // Component logic });
useCallback()
This hook returns a memoized version of the callback function that only changes if one of the dependencies has changed. Useful for passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders.
jsx const memoizedCallback = useCallback(() => { // Callback logic }, [dependencies]);
useMemo()
This hook returns a memoized value. The value is recomputed only when one of the dependencies changes.
jsx const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
The Practice of Windowing: Improving List Performance with react-window Library
Handling large lists or tables can significantly impact performance due to excessive DOM nodes being rendered. The practice of windowing helps address this issue by only rendering visible items within a viewport.
The react-window library provides a set of components for efficiently rendering large lists and tabular data.
Install react-window:
sh npm install react-window
Use a windowed list:
jsx import { FixedSizeList as List } from ‘react-window’;
const Row = ({ index, style }) => (
const MyList = () => ( <List height={150} itemCount={1000} itemSize={35} width={300}
{Row}
Windowing ensures that only a small subset of rows are rendered at any given time, significantly improving performance for large datasets.
Lazy Loading Images for Improved Initial Load Time
Optimizing image loading is essential for enhancing the initial load time of your React application. Implementing lazy loading ensures that images are only loaded when they enter the viewport, thereby reducing the number of HTTP requests during the initial page load.
Implementing Lazy Loading with Intersection Observer API and useEffect Hook
The Intersection Observer API allows you to asynchronously observe changes in the intersection of a target element with an ancestor element or with a top-level document’s viewport. Combining this with the useEffect hook creates an efficient way to handle lazy loading.
Here’s how to implement it:
Set Up the Intersection Observer
javascript import React, { useEffect, useState } from ‘react’;
const LazyImage = ({ src, alt }) => { const [isIntersecting, setIsIntersecting] = useState(false); const imgRef = React.useRef();
useEffect(() => { const observer = new IntersectionObserver( ([entry]) => setIsIntersecting(entry.isIntersecting), { threshold: 0.1 } );
if (imgRef.current) { observer.observe(imgRef.current); } return () => { if (imgRef.current) { observer.unobserve(imgRef.current); } }; }, []);
return ( <img ref={imgRef} src={isIntersecting ? src : ”} alt={alt} style={{ minHeight: ‘200px’, minWidth: ‘300px’ }} /> ); };
export default LazyImage;
Benefits of Lazy Loading
- Reduced Initial Load Time: Only essential resources are loaded initially.
- Improved Performance: Minimizes unnecessary resource downloads.
- Better User Experience: Users see content faster as images load just in time.
Using either method, lazy loading images significantly boosts your application’s performance by deferring off-screen image loading until necessary.
Immutable Data Structures and Web Workers for Enhanced Efficiency
Immutable data structures can significantly improve performance in React applications by preventing unnecessary re-renders. Immutable.js is a popular library that helps manage immutable state effortlessly. By treating data as immutable, developers can:
- Prevent Direct Mutations: Ensure state is not altered directly, which leads to more predictable and maintainable code.
- Enable Efficient Comparisons: Easily determine if changes have occurred by comparing references, speeding up the reconciliation process.
Example:
javascript import { Map } from ‘immutable’;
const state = Map({ count: 0 });
// Instead of mutating the state, return a new instance with updated values const newState = state.set(‘count’, state.get(‘count’) + 1);
console.log(state === newState); // false console.log(state.get(‘count’)); // 0 console.log(newState.get(‘count’)); // 1
Offloading CPU-Intensive Tasks to Web Workers for a More Responsive UI
Web Workers allow you to run scripts in background threads, offloading heavy computations from the main thread. This keeps the UI responsive and improves the overall user experience.
Creating a Web Worker:
Define the Worker Script:
javascript // worker.js self.addEventListener(‘message’, (e) => { const result = performHeavyCalculation(e.data); self.postMessage(result); });
function performHeavyCalculation(data) { // CPU-intensive task here return data * 2; }
Integrate Web Worker in React Component:
javascript import React, { useEffect } from ‘react’;
const MyComponent = () => { useEffect(() => { const worker = new Worker('./worker.js'); worker.postMessage(42); // Sending data to worker worker.addEventListener('message', (e) => { console.log(`Result from worker: ${e.data}`); // Receiving result from worker }); return () => { worker.terminate(); }; }, []);
return Check the console for results; };
export default MyComponent;
By using Immutable.js for state management and implementing Web Workers for CPU-intensive tasks, code React applications can achieve higher efficiency and provide smoother user interactions even during complex operations.
Throttling and Debouncing Events for Better Performance
Efficiently handling events is crucial for maintaining your React application’s performance. Two essential techniques for optimizing event handlers are throttling and debouncing.
What are Throttling and Debouncing?
- Throttling ensures a function is called at most once in a specified time period, regardless of how many times the event is triggered.
- Debouncing delays the execution of a function until after a specified wait time has elapsed since the last time it was invoked.
These techniques help reduce unnecessary function calls, enhancing performance by limiting the frequency at which an event handler runs.
Optimizing Event Handlers with Lodash’s throttle and debounce Functions
Using libraries like Lodash simplifies the implementation of throttling and debouncing. Lodash provides convenient functions to apply these optimizations effortlessly.
Example: Throttling in React
Suppose you have a scroll event in your component that you want to throttle:
jsx import { throttle } from ‘lodash’; import { useEffect } from ‘react’;
const MyComponent = () => { const handleScroll = throttle(() => { console.log(‘Scrolled!’); }, 200);
useEffect(() => {
window.addEventListener(‘scroll’, handleScroll);
return () => {
window.removeEventListener(‘scroll’, handleScroll);
handleScroll.cancel(); // Cancel any pending throttled calls
};
}, []);
return <div>Scroll to see throttled scrolling event</div>;
};
In this example, handleScroll will only be executed once every 200 milliseconds, preventing excessive function calls during continuous scrolling.
Minimizing DOM Operations with React
Minimizing costly DOM operations is essential for boosting the performance of a React application. Excessive and unnecessary manipulations can lead to slow rendering times and a laggy user experience. Below are some techniques to help avoid these pitfalls in your React components:
Techniques for Avoiding Unnecessary DOM Manipulations
Use shouldComponentUpdate or React.memo():
For class components, overriding the shouldComponentUpdate lifecycle method can prevent the component from re-rendering when there are no changes in props or state.
For functional components, React.memo() can wrap the component to ensure it only re-renders when its props change.
jsx const MyComponent = React.memo((props) => { // Component logic });
Batch State Updates:
React batches state updates within event handlers and lifecycle methods, but explicitly batching updates using the unstable_batchedUpdates API can further optimize performance.
jsx import { unstable_batchedUpdates } from ‘react-dom’;
unstable_batchedUpdates(() => { setState1(newState1); setState2(newState2); });
Use Keys for List Rendering:
Ensure each element in a list has a unique and stable key to help React identify which items have changed, been added, or been removed.
jsx {items.map(item => ( ))}
Avoid Inline Functions and Object Creations:
Defining functions or objects inline within JSX can cause unnecessary re-renders as new instances are created on each render.
jsx // Instead of this <button onClick={() => handleClick(item.id)}>Click me
// Do this const handleClick = (id) => () => { /* logic */ };
Click me
Leverage React Fragments:
Use <React.Fragment> or shorthand <> to group multiple elements without adding extra nodes to the DOM, reducing overall DOM node count.
jsx return ( <> </> );
Optimize Conditional Rendering:
Avoid rendering large trees conditionally if they are not needed. Use short-circuit evaluation or ternary operators wisely.
jsx // Instead of this {isConditionMet && }
// Consider this {isConditionMet ? : null}
Debounce Input Handling:
For input fields that require frequent updates, use debouncing techniques to limit the number of renders during user interactions.
By implementing these strategies, you can significantly reduce unnecessary DOM operations and improve your React app’s performance, ensuring a smoother and faster user experience.
Best Practices for State Management in React
Effective state management is crucial for ensuring smooth performance in React applications. Utilizing best practices can significantly reduce unnecessary re-renders and enhance the overall efficiency of your React app performance testing.
Avoiding State Mutations Directly with Immutability Libraries like Immer
Directly mutating state in React can lead to unpredictable behavior and performance issues. Instead, leveraging immutability libraries such as Immer provides a more structured and efficient approach to state management.
Why Use Immutable State?
- Predictability: Immutable data ensures that changes are explicit and trackable.
- Performance: React relies on shallow comparison to detect changes. With immutable data structures, it becomes easier to optimize re-renders.
Using Immer
Immer simplifies the process of working with immutable state by allowing you to “mutate” state directly within a special function called produce. This function produces a new state based on the changes applied.
javascript import produce from ‘immer’;
const initialState = { count: 0, };
const reducer = (state = initialState, action) => { switch (action.type) { case ‘INCREMENT’: return produce(state, draftState => { draftState.count += 1; }); default: return state; } };
In this example, produce takes the current state and a function that applies mutations. These mutations do not alter the original state but instead create a new copy with the updates.
Benefits of Using Immer
- Simplicity: Reduces boilerplate code compared to manually creating new copies of the state.
- Safety: Prevents accidental direct mutations.
- Readability: Keeps code clean and easy to understand.
Utilizing immutability libraries like Immer can profoundly impact your app’s performance, ensuring that state updates are handled efficiently without introducing unnecessary complexity.
Conclusion
Maximizing React app performance is not a one-time task but an ongoing process. Continuous monitoring and optimization ensure that your application remains fast, responsive, and capable of delivering a superior user experience.
Optimizations like throttling and debouncing event handlers, profiling with React DevTools, and configuring Webpack for smaller bundle sizes can significantly impact performance. These methods not only improve load times but also provide a smoother, more engaging experience for users.