Top Facts About React Rendering and Functional Components
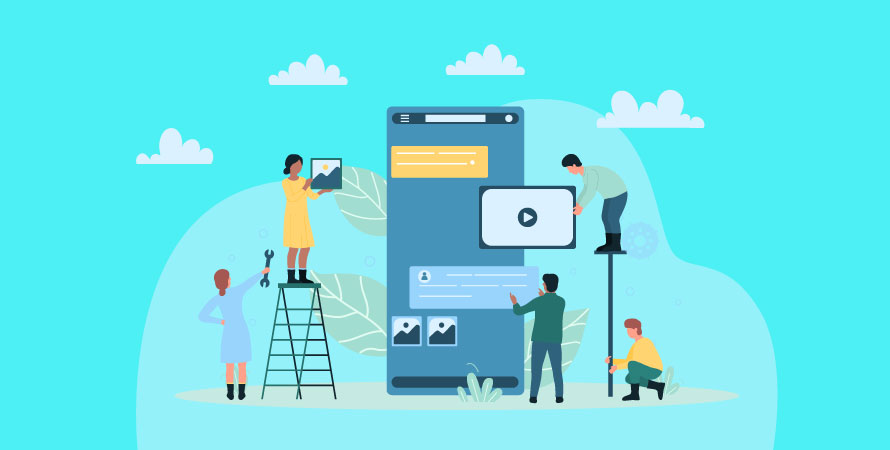
React has changed the way developers build user interfaces with its powerful rendering engine and functional components. React rendering is how React updates the user interface when there are changes in state, props, or context. When a component’s state changes, React re-renders the component and its children to show the new state.
Functional components are an easier way to create components in React. They are JavaScript functions that take props as an argument and return React elements. Unlike class components, functional components don’t have lifecycle methods or local state by default. But with Hooks, functional components can now manage state and side effects just as well.
Understanding how React handles rendering is important for improving performance. If re-renders aren’t managed well, it can lead to slow updates and unresponsive apps. Developers should know how state changes affect rendering and use techniques to minimize unnecessary re-renders.
In this article, we’ll explore interesting facts about React rendering and learn how to optimize functional components for better performance. This React blog will cleary explain the React JS meaning in detail.
Understanding React Rendering
React’s rendering process is central to how it updates and manages the user interface (UI). When a component’s state changes, React re-renders that component to reflect the new state in the UI. This involves creating a new virtual DOM tree and comparing it with the previous one to identify changes. The useeffect in React JS is a React hook that lets you synchronize a component with an external system.
How React Handles State Changes?
When a component’s set state in React changes, it initiates a re-render of that component. This re-rendering process consists of:
- Rendering Phase: The render in React JS method of the component and generates a new virtual DOM tree.
- Diffing Phase: React functional components compare the new virtual DOM tree with the previous one to determine what has changed.
- Commit Phase: The actual updates are applied to the real React DOM based on the differences identified.
Impact on Component and Its Descendants
State changes in a parent component can trigger re-renders for its descendant components. If a parent component re-renders due to a state change, all its child components will also re-render by default. This behavior ensures that the entire subtree reflects the latest state.
Consider an example where a parent component holds a list in its state:
jsx function ParentComponent() { const [items, setItems] = useState([‘Item 1’, ‘Item 2’]);
return ( {items.map((item, index) => ( ))} ); }
Here, updating the items state in ParentComponent will cause both ParentComponent and each ChildComponent to re-render.
Differentiating Between State, Props, and Context Changes
React components can re-render due to changes in:
- State: Local state changes within the component.
- Props: Changes in props passed from parent components.
- Context: Changes in context values provided by React’s Context API.
For instance:
State Change Re-render
jsx function Counter() { const [count, setCount] = useState(0);
return <button onClick={() => setCount(count + 1)}>{count}; }
Clicking the button updates the count state, triggering a re-render of Counter.
Props Change Re-render
jsx function Display({ message }) { return {message}; }
function App() { const [msg, setMsg] = useState(‘Hello’);
return ; }
Updating msg in App will cause Display to re-render with the new prop value.
Context Change Re-render
jsx const ThemeContext = createContext(‘light’);
function ThemedButton() { const theme = useContext(ThemeContext);
return <button className={btn-${theme}}>Themed Button; }
function App() { const [theme, setTheme] = useState(‘light’);
return ( <ThemeContext.Provider value={theme}> </ThemeContext.Provider> ); }
Changing the theme value will trigger ThemedButton to re-render with the updated context value.
Understanding these distinctions helps optimize rendering behavior by pinpointing unnecessary renders and applying appropriate optimization techniques.
Snapshot-based Re-rendering in React
React’s rendering process relies on snapshot-based re-rendering, a technique that ensures the user interface (UI) stays up to date with the latest state. Whenever a state change occurs, React captures a “snapshot” of the UI based on the new state at that moment. It then compares this snapshot with the previous one to determine what has changed.
Understanding Snapshot-based Re-rendering in React
Here’s how snapshot-based re-rendering works in React:
- State Update: When a component’s state changes, React triggers a re-render for that component.
- Snapshot Creation: React generates a new virtual DOM snapshot that represents the current UI based on the updated state.
- Comparison: This new snapshot is compared to the previous one using a process called “diffing” to identify the exact differences.
- Minimal Updates: Only the identified differences between the two snapshots are applied to the actual DOM, ensuring efficient updates without redoing everything.
This approach allows React to maintain high performance even when there are frequent updates by avoiding unnecessary re-renders and focusing only on the specific parts of the UI that require changes.
Using React Devtools to Visualize Component Re-renders
Being able to visualize these component re-renders can be incredibly helpful for optimizing and debugging your React applications. That’s why React Devtools are the smallest building blocks of ReactJS.
Key Features of React Devtools:
- Highlight Updates: Enable highlighting to see which components are updating during state changes.
- Component Tree Inspection: Explore your application’s component tree and inspect props, state, and context for each component.
- Profiler Tab: Specifically designed to help identify performance bottlenecks by showing how long each component took to render.
Steps to Use React Devtools:
Installation:
- If you’re using Chrome, install the React Devtools extension from the Chrome Web Store.
- If you’re using Firefox, install the React Devtools add-on from the Firefox Add-ons website.
Enabling Highlight Updates:
- Open Devtools in your browser (usually by pressing F12 or right-clicking and selecting “Inspect”).
- Go to the Components tab, click on the settings gear icon, and check the option for “Highlight updates when components render”.
Profiling Performance:
- Switch to the Profiler tab within Devtools.
- Start recording interactions by clicking on the “Record” button, perform various actions in your app, and then stop recording to analyze the render times and identify any slow components.
By effectively utilizing these tools, developers can gain deeper insights into how their React applications are rendering and make informed decisions about potential performance optimizations.
Optimizing React Rendering with Pure Components and Memoization
Exploring the Use of Pure Components to Prevent Unnecessary Re-renders
Pure components in ReactJS render are a powerful tool to enhance performance by preventing unnecessary re-renders. A pure component did mount is defined as a component that renders the same output for the same props and state. By implementing React.PureComponent or using the React.memo higher-order component (HOC), React can optimize rendering by performing a shallow comparison of props and state.
Key Points:
- React.PureComponent: Extends React.Component and performs a shallow comparison in shouldComponentUpdate. This reduces re-renders when props and state haven’t changed.
- React.memo: A HOC that memoizes functional components, providing similar benefits as PureComponent. It skips rendering if props remain unchanged between renders.
jsx // Class Component with PureComponent class MyComponent extends React.PureComponent { render() { return {this.props.value}; } }
// Functional Component with React.memo const MyFunctionalComponent = React.memo(({ value }) => { return {value}; });
Implementing Memoization Techniques for Optimized Rendering
Memoization is another technique used to optimize rendering by caching the results of expensive function calls and reusing them when the same inputs occur again. In React advanced concepts, this is commonly achieved using hooks like useMemo and useCallback.
Key Points:
- useMemo: Memoizes the result of a function, recalculating only if dependencies change.
- useCallback: Memoizes a callback function, ensuring it remains stable across renders unless dependencies change.
jsx // Example using useMemo const ExpensiveComponent = ({ count }) => { const calculatedValue = useMemo(() => expensiveCalculation(count), [count]); return {calculatedValue}; };
// Example using useCallback const ParentComponent = () => { const handleClick = useCallback(() => { console.log(‘Button clicked’); }, []);
return ; };
Using these techniques helps avoid unnecessary calculations and improves overall rendering performance. For instance, in scenarios where components receive complex objects or functions as props, memoization ensures that these values are not recreated on every render, thus reducing the workload on the virtual DOM diffing algorithm.
Enhancing performance through pure components and memoization ensures smoother user experiences by minimizing redundant computations and re-renders.
Strategies for Identifying Causes of Component Re-renders in React
Identifying the causes behind component re-renders in React is crucial for maintaining optimal performance. Various techniques and tools can aid developers in pinpointing excessive re-rendering.
Techniques for Detecting Re-renders
Console Logs
Injecting console.log statements within the render method or functional component body can reveal when a component re-renders. This simple technique provides quick insights but might clutter the console during extensive debugging.
javascript const MyComponent = (props) => { console.log(‘MyComponent rendered’); return {props.value}; };
React Profiler
The React Profiler, available in React Developer Tools, offers a powerful way to visualize rendering behaviors. By recording interactions, developers can see which components re-rendered and measure the time taken for each rendering phase.
Why Did You Render (WDYR)
A third-party library designed to detect unnecessary re-renders, WDYR patches React to log information about component renders that might be avoidable.
javascript import whyDidYouRender from ‘@welldone-software/why-did-you-render’; import React from ‘react’;
if (process.env.NODE_ENV === ‘development’) { whyDidYouRender(React, { trackAllPureComponents: true, }); }
const MyComponent = React.memo((props) => { return {props.value}; });
MyComponent.whyDidYouRender = true;
Tools for Analyzing Rendering Behavior
- React Developer Tools: This browser extension not only includes the Profiler but also offers insights into props and state changes across components, helping identify the root cause of re-renders.
- Performance Tab in Chrome DevTools: By recording performance profiles, developers can analyze scripting and rendering times to understand how different components contribute to overall app performance.
Best Practices to Optimize React Components’ Rendering Performance
Improving rendering performance in React applications is essential for delivering a smooth and responsive user experience. Here are some key tips for optimizing the rendering of your React components:
1. Use React.memo
React.memo is a higher-order component that memoizes the result of a functional component’s render, preventing unnecessary re-renders when the props haven’t changed.
jsx const MyComponent = React.memo((props) => { // Component logic });
2. Avoid Inline Functions in Render
Inline functions cause new references to be created on each render, leading to avoidable re-renders. Move functions outside of the render method.
jsx // Inefficient const MyComponent = () => ( <button onClick={() => console.log(‘Clicked’)}>Click me );
// Efficient const handleClick = () => console.log(‘Clicked’); const MyComponent = () => ( Click me );
3. Split Large Components
Breaking down large components into smaller, reusable components helps manage re-renders more efficiently.
jsx function ParentComponent() { return ( <> </> ); }
4. Implement Pure Components
A pure component only re-renders when its props or state change. Using React.PureComponent ensures shallow comparison of props and state.
jsx class MyPureComponent extends React.PureComponent { render() { return {this.props.value}; } }
5. Use useCallback and useMemo Hooks
The useCallback hook memoizes callback functions, while useMemo memoizes values, both reducing unnecessary re-renders.
jsx const MyComponent = ({ value }) => { const memoizedValue = useMemo(() => computeExpensiveValue(value), [value]);
const handleClick = useCallback(() => { // Function logic }, []);
return {memoizedValue}; };
6. Optimize Context Usage
Frequent updates in context can lead to excessive re-renders. Use context wisely by splitting into multiple contexts if needed.
jsx const UserContext = React.createContext(); const ThemeContext = React.createContext(); // Separate contexts for different data needs.
7. Lazy Load Components
Lazy loading components with React.lazy and Suspense ensures that only necessary components are loaded initially, improving load time and rendering performance.
jsx const LazyComponent = React.lazy(() => import(‘./LazyComponent’));
function App() { return ( <React.Suspense fallback={Loading…}> </React.Suspense> ); }
These practices help ensure your React applications remain performant and responsive under varying conditions and loads. Employing these techniques can significantly optimize rendering behavior, leading to better overall user experiences.
Advanced Concepts and Techniques in React Rendering
To improve the performance of React fibre applications, it’s important to explore advanced rendering concepts such as concurrent rendering and render batching.
Concurrent Rendering
Concurrent rendering enables React to work on multiple versions of the UI at the same time. This is crucial for handling complex user interfaces that require quick responses. Instead of getting stuck on long render calculations, concurrent rendering divides tasks into smaller parts, allowing React hooks list to pause and resume work as necessary. As a result, users experience smoother interactions even when there’s heavy activity.
Render Batching
Render batching is another effective optimization technique. Rather than immediately processing each state update and triggering a re-render, React combines multiple updates together into a batch. This reduces the number of renders and significantly improves performance. By grouping updates, React can minimize the extra work involved in updating the DOM and ensure smoother transitions between UI states. In this way, React can render more hooks than during the previous render.
Introducing Concurrent Mode
React’s concurrent mode builds upon these concepts to enhance the user experience. When concurrent mode is enabled:
- Urgent updates are prioritized: User actions like typing or clicking are given higher priority than less important tasks.
- Work can be interrupted and resumed: Lengthy tasks can be paused and resumed, ensuring that the UI remains responsive.
- Multiple tasks can be handled concurrently: Background tasks are executed without blocking the main thread.
Concurrent mode introduces several new APIs and patterns that leverage these capabilities:
- useTransition: Allows developers to mark certain state updates as non-urgent, enabling smoother transitions.
- Suspense: Pauses updates until a resource is ready (e.g., data fetching), ensuring consistent UI states.
Handling Edge Cases for Optimal React Rendering
Optimizing React rendering performance involves identifying and addressing edge cases that can cause inefficiencies. These situations, though uncommon, can significantly impact the responsiveness and speed of your application.
Identifying Common Edge Cases
Frequent State Updates
Rapidly changing state values can trigger excessive re-renders. For instance, continuously updating a counter or fetching data in quick succession can lead to performance bottlenecks.
jsx const [count, setCount] = useState(0);
useEffect(() => { const interval = setInterval(() => setCount(prev => prev + 1), 100); return () => clearInterval(interval); }, []);
Deeply Nested Components
State changes in deeply nested components can cascade upwards, causing multiple re-renders across the component tree.
Large Lists
Rendering large lists without optimization techniques like virtualization can degrade performance due to the sheer number of DOM elements being manipulated.
Strategies for Addressing Edge Cases
Debouncing State Updates
Implement debouncing to limit how frequently state updates occur, thus reducing the number of re-renders.
jsx const handleInputChange = debounce((value) => { setState(value); }, 300);
Memoization
Use React.memo for functional components and React.PureComponent for class components to prevent unnecessary re-renders when props remain unchanged.
jsx const MemoizedComponent = React.memo(MyComponent);
Virtualization for Large Lists
Libraries like react-window or react-virtualized help manage large lists efficiently by only rendering visible items.
jsx import { FixedSizeList as List } from ‘react-window’;
Measuring and Monitoring Performance
Utilize tools like React DevTools Profiler to track component render times and identify performance bottlenecks:
- React DevTools: Helps visualize component trees and detect unnecessary re-renders.
- Chrome DevTools: Provides detailed insights into JavaScript execution and rendering performance.
By proactively identifying and addressing these edge cases, you can ensure a smoother, more efficient render method in your React applications.
Additional Considerations for Fine-tuning React Rendering Behavior
Understanding the Role of DOM Manipulation After Component Rendering
After a component renders, Reactdom render takes charge of manipulating the DOM to reflect the current UI state. This process involves:
- Minimal Updates: ReactJS set state ensures only necessary changes are made to the DOM, reducing performance overhead.
- Batching Updates: Multiple updates are batched together to optimize rendering performance.
Direct DOM manipulation outside of React’s control can lead to discrepancies between the actual DOM and React’s virtual DOM, potentially causing unexpected behavior and rendering issues.
Evaluating the Impact of Asynchronous State Updates on Rendering Stability
Asynchronous state updates can significantly influence React render method. Key points to consider include:
- State Queueing: React createelement maintains a queue for state updates, applying them in batches during re-renders.
- Component Consistency: Asynchronous updates ensure components remain consistent with the application state without blocking user interactions.
- Potential Pitfalls: Improper handling of async state updates may lead to race conditions or stale closures, where components rely on outdated state values.
To handle asynchronous updates effectively, leveraging hooks like useEffect and ensuring proper dependency management is crucial.
Utilizing Refs to Measure Dimensions of DOM Nodes Accurately
Refs provide a way to access DOM nodes directly within functional components. They are particularly useful for:
- Measuring Dimensions: Using refs to measure widths, heights, or positions of elements in relation to layout adjustments.
- Avoiding Re-renders: Unlike state changes that trigger re-renders, updating refs does not cause re-rendering, preserving performance.
- Dynamic Adjustments: Implementing dynamic layout adjustments based on real-time measurements from refs.
React useeffect example usage: jsx const MyComponent = () => { const myRef = useRef(null);
useEffect(() => { const dimensions = myRef.current.getBoundingClientRect(); console.log(dimensions.height); // Access height }, []);
return Measure me!; };
Incorporating these additional considerations into your React development workflow can lead to more stable and efficient applications.
Conclusion
Mastering React rendering optimization is a journey that involves understanding how React handles UI updates. By grasping the core principles of state changes, snapshot-based re-rendering, and the role of the virtual DOM, developers can significantly enhance the performance of their applications.
Optimizing React rendering requires both theoretical knowledge and practical application. With a solid understanding of these concepts, developers can build highly efficient, responsive applications that provide an optimal user experience.