The Secrets to Creating Amazing SPAs Using React JS
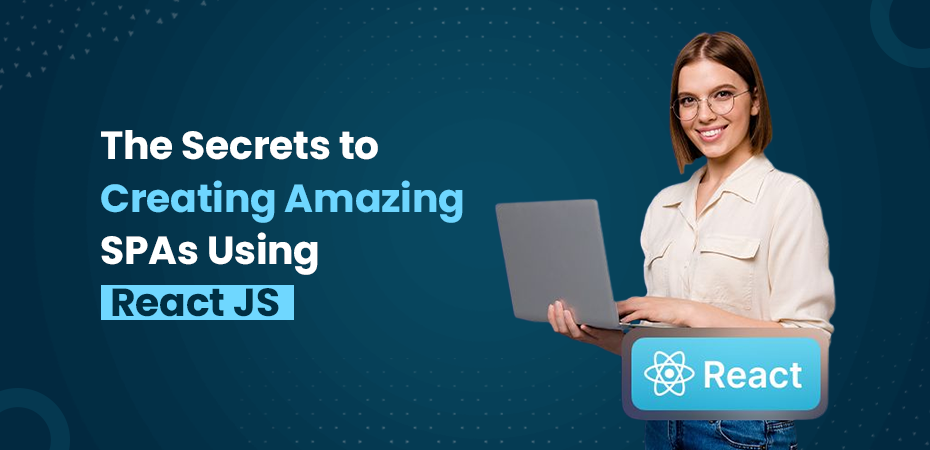
A Single Page Application (SPA) is a web application that loads a single HTML page and dynamically updates that page as the user interacts with the app. This approach contrasts with traditional multi-page applications, where each user action requires loading a new page from the server.
Key characteristics of SPAs include:
- Dynamic Content Loading: SPA apps fetch data and content in the background, updating the current page without requiring a full reload. This process involves creating a React register and login single page app.
- Enhanced User Experience: By avoiding full-page reloads, single-page web apps provide a smoother and faster user experience.
- JavaScript-Centric: One-page app building heavily relies on JavaScript frameworks/libraries like React, Angular, or Vue.js for building interactive UIs.
Dynamic Page Rewriting vs. Traditional Page Loading
In traditional multi-page applications, each interaction like clicking a link or submitting a form results in a request to the server page. The question arises can a user add content to a React app?
By analyzing this, the server responds by sending an entirely new HTML page, causing the browser to refresh. This process can be slow and disrupts the user experience.
SPAs, on the other hand, use dynamic page rewriting. Here’s how it works:
- Initial Load: In a one-page web app building, the browser loads a single HTML page along with CSS and JavaScript files.
- User Interaction: When users interact with the app (e.g., clicking links or buttons), JavaScript intercepts these actions.
- Data Fetching: JavaScript fetches only the required data from the server using APIs (e.g., RESTful APIs or GraphQL).
- DOM Manipulation: The fetched data is used to update parts of the Document Object Model (DOM) without reloading the entire page.
Example:
javascript // Fetching data using JavaScript fetch(‘/api/data’) .then(response => response.json()) .then(data => { // Update DOM dynamically document.getElementById(‘content’).innerHTML = renderContent(data); });
This method ensures quicker interactions and less bandwidth usage.
User Experience Benefits of SPAs
The dynamic nature of React SPA offers several significant benefits to user experience:
- Speed and Performance: Since only necessary data is fetched and rendered, interactions are much faster compared to traditional apps.
- Seamless Navigation: In a React single-page app, users enjoy smooth transitions between different views or sections without experiencing disruptive page reloads.
- Rich Interactivity: The React single-page applications have enhanced interactivity through real-time updates, animations, and more responsive interfaces.
Consider popular web applications like Facebook or Twitter. These platforms utilize SPA principles to allow users to scroll through feeds, load new content seamlessly, and interact with posts without ever experiencing a full-page refresh.
In summary, understanding what makes up a JS SPA provides insight into its advantages over traditional web applications.
Single Page Applications vs Multi Page Applications
Key Differences Between SPAs and MPAs
When comparing Single Page Applications (SPAs) with Multi Page Applications (MPAs), several distinguishing features emerge:
1. Rendering Approach
- SPAs: Load a single HTML page and dynamically update content as the user interacts with the application. Hence, learning about single-page apps meaning minimizes full-page reloads.
- MPAs: The React JS simple app consists of multiple HTML pages, each one being loaded separately from the server upon navigation.
2. User Experience
- SPAs: Offer a smoother, more fluid experience with faster interactions since only parts of the page are updated.
- MPAs: Can feel slower as each user action that requires new data leads to a full page reload.
3. Development Complexity
- SPAs: Typically involve more complex JavaScript code to handle dynamic content updating.
- MPAs: SPAs are simpler in terms of front-end development and involves more server-side logic to handle different pages.
Advantages and Disadvantages
Advantages of SPAs
- Performance: The React one-page app involves faster loading times after the initial load, as subsequent interactions require less data transfer.
- User Experience: Javascript single-page applications comprise seamless navigation and interaction without page reloads.
- Reduced Server Load: Fewer requests are sent to the server after the initial load on the React site.
Disadvantages of SPAs
- SEO Challenges: Harder to optimize for search engines because of dynamic content loading.
- Initial Load Time: The single-page javascript application can be slower initially as all necessary assets must be downloaded upfront.
- Browser Compatibility Issues: A sample React JS app may require additional handling for older browsers.
Advantages of MPAs
- SEO Friendliness: Each page can be individually optimized for search engines.
- Progressive Enhancement: Easier to implement fallbacks for older browsers or users with JavaScript disabled.
- Scalability in Development Teams: Easier to divide work among multiple teams focusing on different pages.
Disadvantages of MPAs
- Performance Lag: Slower user interactions due to full-page reloads on every navigation.
- Higher Server Load: A single-page application template includes more frequent server requests leading to potential performance bottlenecks.
Top Scenarios for Choosing SPAs Over Multi-Page Applications
Choosing between an SPA or MPA largely depends on the specific requirements and goals of your project:
- Data-Rich Applications: SPAs excel in applications requiring real-time data updates, such as dashboards, where seamless updates enhance usability.
- Mobile-first Experiences: For mobile users, the quick interactions provided by SPAs can significantly improve user satisfaction.
- Interactive User Interfaces: One-page application examples include social media platforms (e.g., Facebook). These benefit from SPA’s ability to provide instantaneous feedback without disrupting the user experience.
- Content-centric Websites: MPAs might be a better fit for websites focused on static content or where SEO is crucial, such as blogs or news sites.
- E-commerce Sites: Often benefit from MPAs as of better individual page optimization for search engines, crucial for product discovery and sales conversions.
Understanding these differences helps developers make informed decisions about which architecture best suits their project’s needs.
Advantages of SPAs
Enhanced Performance and Faster Interactions
Single Page Applications (SPAs) offer enhanced performance by loading a single HTML page and dynamically updating content as the user interacts with the app. This reduces the need for multiple server requests, leading to faster interactions. Instead of reloading entire pages, SPAs only transfer necessary data, resulting in a more efficient user experience.
Improved User Experience Through Seamless Navigation
The hallmark of SPAs is their ability to provide a seamless navigation experience. Users can interact with the application without experiencing page reloads or delays, which is crucial for maintaining engagement. By dynamically updating content, SPAs mimic the behavior of desktop applications, offering a smooth and intuitive user interface.
Reduced Server Load and Bandwidth Usage
SPAs significantly reduce server load and bandwidth usage. Because only the required data is fetched and rendered on the client side, the amount of data transmitted between client and server is minimized. This leads to lower latency and faster load times, contributing to an overall better performance.
Simpler Testing and Maintenance Processes
Testing and maintaining SPAs can be simpler compared to traditional multi-page applications (MPAs). The component-based architecture of frameworks like React allows developers to isolate parts of the application for testing. This modular approach not only facilitates easier debugging but also makes it straightforward to update or maintain specific components without affecting the entire application.
By using these advantages, SPAs present a compelling choice for modern web development, especially when creating dynamic and highly interactive applications that prioritize user experience and performance.
Steps for Creating Single Page Applications with React
1. Setting Up the Development Environment
To begin your journey into React JS development and learn how to build SPA using React JS, the first step in creating a simple React app involves setting up your development environment:
Tools needed for setup
- Node.js: Ensure Node.js is installed on your machine. It provides the runtime environment for JavaScript and includes npm (Node Package Manager).
- Code Editor: Choose a code editor that supports React development, such as Visual Studio Code, Sublime Text, or Atom.
Using Create React App for project initialization
- bash npx create-react-app my-spa cd my-spa npm start
- Create React App simplifies the initial configuration by providing a ready-to-use template.
2.Installing Necessary Packages
Efficient package management is crucial in modern web development:
Importance of npm for package management
- Npm allows you to install, share, and manage dependencies required for your project.
Installing essential libraries like react-router-dom
- bash npm install react-router-dom
- This library is indispensable for handling routing within your SPA.
3. Creating Components
Building reusable components is a core principle of React:
Main application component (App.js)
- The heart of your SPA where other components will be rendered.
Additional components for different views
- Home.js
- About.js
Example of a simple component:
jsx import React from ‘react’;
const Home = () => { return ( Home Welcome to the home page! ); };
export default Home;
4. Implementing Routing
Routing is essential for navigation in SPAs:
Setting up routes with React Router
- jsx import { BrowserRouter as Router, Route, Switch } from ‘react-router-dom’;
- function App() { return ( ); }
- export default App;
Managing navigation links with <NavLink>
- jsx import { NavLink } from ‘react-router-dom’;
- const Navigation = () => ( Home About );
- export default Navigation;
5. State Management Techniques
Effectively managing state ensures a responsive and dynamic app:
Overview of state management in React apps
- Utilize built-in hooks like useState and useContext for simpler state needs.
Using external libraries like Redux
- For complex state management scenarios, Redux offers robust solutions.
- Example using useState:
- jsx import React, { useState } from ‘react’;
- const Counter = () => { const [count, setCount] = useState(0);
- return (
- <div>
- <p>You clicked {count} times</p>
- <button onClick={() => setCount(count + 1)}>
- Click me
- </button>
- </div>
- );
- };
- export default Counter;
6. Handling API Calls
Dynamic data fetching enhances user interaction:
- Using JavaScript’s fetch API or Axios library:
- jsx import axios from ‘axios’;
- const fetchData = async () => { const response = await axios.get(‘https://api.example.com/data’); console.log(response.data); };
- fetchData();
7. Performance Optimization Techniques
Optimizing performance is vital for a smooth user experience:
- Code splitting and lazy loading:
- jsx import React, { Suspense, lazy } from ‘react’;
- const LazyComponent = lazy(() => import(‘./LazyComponent’));
- function App() { return ( <Suspense fallback={Loading…}> ); }
- export default App;
8. Testing and Deployment Practices
Testing ensures reliability before going live:
- Use testing frameworks like Jest and React Testing Library.
- Example test using Jest:
- jsx import { render } from ‘@testing-library/react’; import App from ‘./App’;
- test(‘renders without crashing’, () => { render(); });
Deploy using services like Vercel or Netlify for seamless integration.
9. SEO Considerations for SPAs
Improving SEO can be challenging but essential:
- Use server-side rendering (SSR) with frameworks like Next.js.
- Implement meta tags dynamically using libraries like react-helmet.
By following these steps, you can master how to build SPA using React JS and create efficient, high-performance applications.
Some Examples of Successful Single Page Applications
These SPA application examples show how effectively they can enhance user experiences. Here are some notable examples:
Facebook’s web application exemplifies the power of SPAs. Users can interact with various features like news feeds, messages, and notifications without experiencing full page reloads. This seamless interaction is enabled by dynamic content updates, ensuring a fluid user experience.
- Use Case: Social networking platform.
- Features: Real-time updates, instant messaging, dynamic news feed.
- Capabilities: This platform has enhanced performance through asynchronous data fetching. This reduces server load causing fewer full-page requests.
Twitter utilizes an SPA approach to offer real-time updates and interactions. As users scroll through their feed or click on tweets, content dynamically refreshes without a complete page reload.
- Use Case: Microblogging and social networking service.
- Features: Infinite scrolling, instant notifications, tweet composition.
- Capabilities: Efficient data management with AJAX calls, improved user engagement through quick interactions.
Netflix
Netflix’s streaming service is another prime example of a successful SPA. Users can browse through various categories, search for content, and watch trailers without leaving the current page. The player loads instantaneously while switching between episodes or movies.
- Use Case: Video streaming platform.
- Features: Dynamic content loading, personalized recommendations, seamless video playback.
- Capabilities: High performance with lazy loading techniques, efficient state management for user preferences.
These examples illustrate the practical applications of SPAs in delivering fast and responsive web experiences. They showcase how leveraging technologies like React JS can transform traditional web applications into dynamic and engaging platforms.
Conclusion
Starting your journey to build SPAs with React JS offers developers a roadmap to create efficient and dynamic single-page applications. By setting up your environment, installing essential packages, and implementing optimization techniques, you’re well-equipped to build engaging SPAs.
Experimenting with components, routing, and state management helps refine your projects. As React JS evolves, the potential for creating powerful SPAs grows, positioning you at the forefront of web development and preparing you for future innovations and challenges.