Testing React Apps: A Comprehensive Guide for Beginners
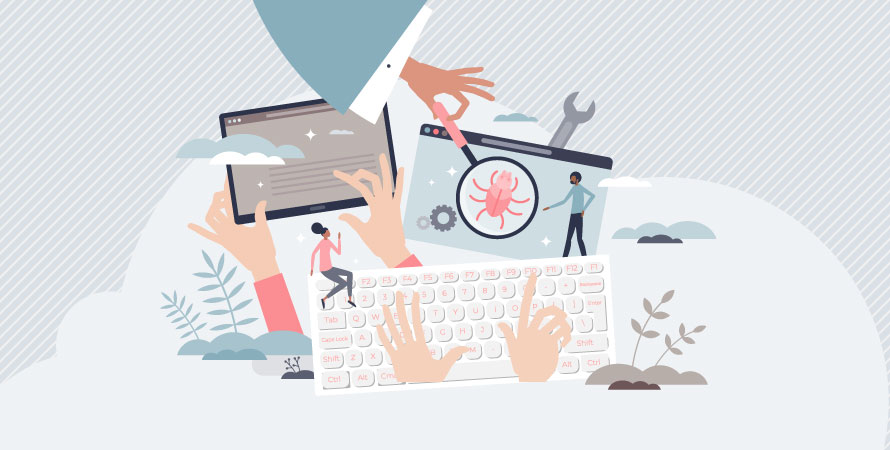
Testing is a critical phase in software development, ensuring that code meets its requirements and behaves as expected. For developers working with React web applications, a popular JavaScript library for building user interfaces, adopting a robust testing strategy is vital to deliver high-quality applications. This comprehensive guide aims to equip beginners with the foundational knowledge and practical skills needed for testing React apps.
React Testing Library (RTL) offers a lightweight solution for testing components by mirroring how users interact with the application. It provides utility functions that help in querying the DOM in a maintainable and accessible way. Coupled with Jest, a JavaScript test runner that supports unit and snapshot testing, these tools create a powerful combination for React developers.
The benefits of testing Reactjs apps are manifold:
- Detecting Bugs Early: Automated tests can uncover issues before they reach production.
- Simplifying Refactors: Tests act as safeguards when modifying code, ensuring changes don’t break existing functionality.
- Improving Code Quality: Writing tests encourages better coding practices and more maintainable code structures.
- Documentation: Tests serve as living documentation for how components should behave.
Embarking on this journey of learning to test React apps will not only enhance the quality of your applications but also sharpen your development skills for future projects.
Understanding React Testing Library
React Testing Library (RTL) is a powerful framework for testing the DOM in React. It provides a wide range of tools to validate different aspects of React applications. RTL focuses on tests that closely resemble how users interact with your components, making sure that the test environment mimics real-world usage as much as possible.
Writing Your First Test with React Testing Library
To start writing tests with React Testing Library, it’s important to understand its core principles. Instead of focusing on implementation details, tests should prioritize the functionality that is visible to the user. With this approach, let’s look at how to create a basic test.
Step 1: Create a Component to Test
First, you’ll have a component that you want to test. Let’s consider this simple Greeting component:
jsx function Greeting({ name }) { return Hello, {name}!; }
Step 2: Write the Test Using RTL
A test for this component using RTL would look like this:
javascript // Importing utilities from RTL and the component to be tested import { render, screen } from ‘@testing-library/react’; import Greeting from ‘./Greeting’;
test(‘it greets the user by name’, () => { // Render the component with props render();
// Assert that the expected outcome is in the document expect(screen.getByText(‘Hello, Jess!’)).toBeInTheDocument(); });
Here’s what happens in this test case:
- render(): This function takes your React app js component and mounts it within a virtual DOM provided by Jest.
- screen: A utility provided by RTL for querying DOM elements. It effectively represents what’s rendered on the page.
- getByText: A query method used to find elements based on their text content.
- expect: Part of Jest’s assertion library, it’s used to define expectations about what should be true in the test environment.
- toBeInTheDocument(): A matcher that checks if an element is present in the virtual DOM.
Basic Structure of a Test Case in React Testing Library
Here, you will know how to check if you have react installed properly. When structuring a test case in RTL, you typically follow the Arrange-Act-Assert pattern:
- Arrange: Set up the conditions required for your test. This includes rendering your component within RTL’s virtual DOM environment.
- Act: Execute actions that mimic user behavior or trigger state changes within your component.
- Assert: Check that the outcome matches your expectations.
This structure ensures tests are organized and maintainable, clearly outlining each stage of interaction with your components.
Common Queries and Matchers for Selecting DOM Elements in Tests
RTL offers various queries to select elements within the virtual DOM for assertions. These include:
- getBy: Returns the first matching node for a query, and throws an error if no elements match or if more than one match is found.
- getAllBy: Returns an array of all matching nodes for a query, and throws an error if no elements are found.
- queryBy: Like getBy, but returns null when no elements match instead of throwing an error.
- queryAllBy: Like getAllBy, but returns an empty array when no elements are found instead of throwing an error.
- findBy: Returns a Promise which resolves when an element is found which matches the given query. Useful for asynchronous queries.
For each type of query (get, getAll, query, etc.), there are multiple methods available depending on what you need to select:
- ByText: Selects elements based on their text content.
- ByPlaceholderText: Finds an input element based on its placeholder value.
- ByLabelText: Selects form elements associated with a label via text content.
- ByAltText: Looks for images based on their alt attribute.
- ByTestId: Targets elements by their data-testid attribute, which is useful when there is no semantic query available.
Matchers are used alongside these queries to write assertions about nodes:
- .toBeInTheDocument()
- .toBeVisible()
- .toHaveClass()
- .toHaveAttribute()
- .toHaveTextContent()
These assertions help verify properties of selected nodes, such as visibility or text content, allowing developers to ensure their components behave as expected under specific conditions.
Testing React Components with RTL
React components are the building blocks of any React application. They encapsulate functionality and rendering logic, making it crucial to ensure they work as intended. React Testing Library provides a robust set of tools for DOM testing that enable developers to write tests that closely mimic how users interact with the application.
Key Features and Principles of React Testing Library
- Focuses on testing components from the user’s perspective.
- Encourages the use of accessible queries, thereby promoting better accessibility practices.
- Avoids testing implementation details, such as component state or lifecycle methods.
- Provides utilities for firing events to simulate user actions.
Advantages of Using React Testing Library to Testing React JS Apps
- Enhances confidence in the application by ensuring components respond correctly to user input.
- Improves maintainability by avoiding reliance on internal APIs or structure.
- Streamlines the development process with fast feedback loops.
Simulating User Interactions
To simulate user interactions, developers use methods like fireEvent or the more comprehensive userEvent library which wraps around fireEvent. These functions simulate real browser events, including mouse clicks, keyboard input, and form submissions. For example:
javascript import { render, fireEvent } from ‘@testing-library/react’; import MyButton from ‘./MyButton’;
test(‘button click increments counter’, () => { const { getByText } = render(); const button = getByText(/click me/i);
// Simulate a user clicking the button fireEvent.click(button);
// Assert the expected outcome after interaction expect(getByText(/clicked: 1 times/)).toBeInTheDocument(); });
In this snippet, a button is rendered using the render method from RTL. The fireEvent.click simulates a user clicking on it and afterward, an assertion is made to confirm that the button’s click count has incremented.
Asserting Component Behavior
Assertions are pivotal in verifying that a component behaves as expected after specific interactions or lifecycle events. RTL integrates seamlessly with Jest matchers allowing robust assertions about the DOM elements.
javascript import { render } from ‘@testing-library/react’; import UserGreeting from ‘./UserGreeting’;
test(‘renders a greeting message when loaded’, () => { const { getByText } = render();
// Asserts that the greeting message appears on screen after rendering expect(getByText(/hello, user!/i)).toBeInTheDocument(); });
Here, after rendering a UserGreeting component, we assert whether a greeting message is part of the document—ensuring that our UI renders what users should see.
By applying these methods for simulating events and asserting conditions in React components, developers leverage React Testing Library to create meaningful tests that contribute positively to app stability and user experience. With testing components through RTL’s straightforward approach, one can validate not just presence but also functionality—emphasizing behavior over implementation details.
Jest Framework for Testing in React
The Jest framework is essential for testing in the React ecosystem. It offers great features such as easy setup, snapshot testing, and built-in test runners. This makes Jest the top choice for developers who want to ensure their applications are bug-free and maintain high-quality standards.
Writing Unit Tests with Jest
Unit testing is crucial for a strong testing strategy. It involves testing individual pieces of code, usually functions or classes, separately from the rest of the application. Here’s how you can start writing unit tests using the Jest framework:
Setting up Jest
Before you can write ReactJS exams, you need to set up Jest in your React project:
- Install Jest using npm or yarn: bash npm install –save-dev jest
- or bash yarn add –dev jest
- Add a script to your package.json to run your tests: json “scripts”: { “test”: “jest” }
- Create a test file next to the file that you are testing. By convention, it is named as filename.test.js.
Writing Your First Unit Test
To create a React App test in Jest, follow these steps:
- Define a test block using it or test.
- Provide a description of what the test should do.
- Write your assertions within the block.
Here’s an example:
javascript // sum.js const sum = (a, b) => a + b;
module.exports = sum;
// sum.test.js const sum = require(‘./sum’);
test(‘adds 1 + 2 to equal 3’, () => { expect(sum(1, 2)).toBe(3); });
In this example:
- test is the function used to define a test case.
- ‘adds 1 + 2 to equal 3’ is the description of what the test does.
- expect is a function provided by Jest to check that a value meets certain conditions – these are called matchers.
Matchers
Jest provides a variety of matchers that allow you to write clear tests:
- .toBe(value) checks strict equality.
- .toEqual(value) checks value equality and works well for arrays and objects.
- .toContain(item) ensures an array contains a specific item.
For more complex assertions, you can use matchers like .toThrow() for functions that should throw errors or .toMatchObject(object) for checking if an object matches a subset of properties of another object.
Testing Pure Functions and Utility Modules with Jest
Pure functions are ideal for unit testing because they don’t rely on external state or produce side effects. They take inputs and return outputs predictably.
Example: Testing a Pure Function
Let’s say we have a utility module with pure functions used throughout our application:
javascript // utils.js export const capitalize = (string) => string.charAt(0).toUpperCase() + string.slice(1);
export const reverseString = (string) => string.split(”).reverse().join(”);
// utils.test.js import { capitalize, reverse string } from ‘./utils’;
test(‘capitalize capitalizes the first letter of a string’, () => { expect(capitalize(‘react’)).toBe(‘React’); });
test(‘reverse string reverses the string’, () => { expect(reverse string(‘jest’)).toBe(‘tsej’); });
In these examples:
- The capitalize function takes a string and returns it with its first letter capitalized.
- The reverseString function takes a string and returns it reversed.
- We test each function with inputs we know the output for.
By focusing on pure functions that do not rely on external systems or states, we solidify our confidence in their reliability through Jest’s straightforward assertions.
When transitioning from unit testing individual functions to examining how components interact with each other through integration testing, one might encounter complexities due to dependencies between components. This challenge calls for approaches that may include mocking dependencies or utilizing shallow rendering techniques.
As we move forward with our exploration of testing React apps, we’ll delve into how Jest enables integration testing and what practices can be adopted for effectively simulating component interaction without introducing external variables into our test environment.
Different Types of Tests in React Apps
ReactJS testing framework involves several levels of tests, each serving its purpose in the development lifecycle. The Test Pyramid represents the ideal balance and quantity of each test type, advocating for a greater number of low-level unit tests and fewer high-level end-to-end tests.
Unit Testing
In the context of React native apps, unit testing refers to the practice of testing individual parts of the code in isolation from the rest of the application. These “units” can be functions, components, or hooks that are tested to ensure they work as intended.
- Pure Functions: For functions that return values based solely on their inputs without side effects, assertions check whether the output matches expectations.
- Components: When testing React components, it’s about ensuring that given specific props or states, the component renders correctly. Libraries like Jest provide utilities for shallow rendering to facilitate this.
- Hooks: Custom hooks can also undergo unit testing by invoking them within a test environment and monitoring their return values and state changes over time.
Integration Testing
Integration testing assesses how well different parts of the application work together. In React, this could mean testing a component along with its child components or ensuring that various hooks and context providers function cohesively.
- Component Interaction: Verify that parent and child components communicate properly when integrated, passing props and updating states as expected.
- Context & Hooks: Test the interaction between different hooks and context providers to ensure state is managed accurately across different parts of your app.
Component Testing
Component testing is somewhat of a hybrid between unit and integration tests. It focuses on an individual component but in more depth than a basic unit test, often involving user interaction and lifecycle methods.
- Stateful Components: Validate that components correctly initialize and update their state in response to user actions or API responses.
- Event Handling: Simulate events like clicks or input changes to verify that event handlers are triggered correctly and produce the desired outcomes.
End-to-End Tests
Moving up the pyramid, end-to-end tests serve as a comprehensive method for validating the entire application’s flow—simulating real user scenarios from start to finish.
- User Flows: Follow common user paths through your application to ensure all steps function together seamlessly.
- Network Calls: Simulate interactions with backend services to validate both success and error-handling scenarios within your app’s UI.
By covering each level with appropriate tests—unit for isolated functionality, integration for module collaboration, component for a thorough inspection of individual units, and end-to-end for a full user experience simulation—developers create robust applications less prone to regressions. As you progress further into testing React applications with Jest and React Testing Library, keeping these types at hand ensures thorough coverage for reliable software delivery. Moving forward, let’s explore best practices for leveraging these test types effectively.
Best Practices for Effective Testing with React Testing Library and Jest
Adopting React app testing best practices and Jest best practices plays a crucial role in ensuring the quality, reliability, and maintainability of React applications. The following guidelines are instrumental for developers seeking to enhance their testing strategies.
Writing Readable and Maintainable Tests
Use Descriptive Test Names
Choose test names that clearly describe what they are verifying. Descriptive test names function as documentation and make it easier to identify tests that need attention when they fail.
javascript // Good test(‘renders the user profile link’, () => { // Test content });
// Avoid test(‘test1’, () => { // Test content });
Arrange-Act-Assert Pattern
Organize your tests by separating the setup (arrange), the execution of the functionality to be tested (act), and the assertions (assert). This structure promotes readability and helps others understand the flow of your tests.
javascript test(‘increments counter’, () => { // Arrange const { getByTestId } = render();
// Act fireEvent.click(getByTestId(‘increment-button’));
// Assert expect(getByTestId(‘counter’)).toHaveTextContent(‘1’); });
Keep Tests DRY but Not Too DRY
While it’s important to avoid code duplication, creating overly abstracted tests can reduce clarity. Aim for a balance where common setup or utilities are extracted, but tests remain explicit about their intentions.
Comment Wisely
Use comments to explain why certain actions are taken within tests if it’s not immediately obvious from the code itself. Avoid redundant comments that simply restate what the code does.
Mocking External Modules and API Requests
Mock Dependencies Selectively
Mock external dependencies when their internal workings are irrelevant to the behavior under test. This isolates your test subject and ensures your tests run quickly, without actual network delays or side effects.
javascript jest.mock(‘../api’); // Mock an entire module
// Mock a specific implementation jest.mock(‘../api’, () => ({ fetchUserData: jest.fn(() => Promise.resolve({ name: ‘Alice’ })), }));
Simulate Realistic Scenarios with Mocks
When mocking functions or API requests, provide realistic data that closely resembles what your components expect in real use cases. This helps ensure your components will behave correctly with actual data.
javascript // Mock a successful API request with realistic response data axios.get.mockResolvedValue({ data: { id: 1, title: ‘Test Post’, content: ‘Lorem ipsum dolor sit amet…’ } });
Restore Original Implementations When Needed
After mocking functions or modules for specific tests, restore them to their original implementations if later tests require the actual functionality.
javascript afterEach(() => { jest.restoreAllMocks(); });
By embracing these best practices in app js react testing with React Testing Library and Jest, developers create a robust foundation for reliable automated testing. With readable and maintainable tests complemented by thoughtful mocking strategies, teams can build confidence in their applications’ correctness and long-term quality.
Conclusion
Testing React applications may seem overwhelming at first, but with the knowledge gained from this guide, beginners can confidently use React Testing Library and Jest to create robust and reliable applications.
Testing should not be an afterthought but an integral part of the development process. By investing time in writing thorough tests, you can ensure that your React apps perform flawlessly for every user.