ReactJS vs AngularJS: Which is Better for 2024?
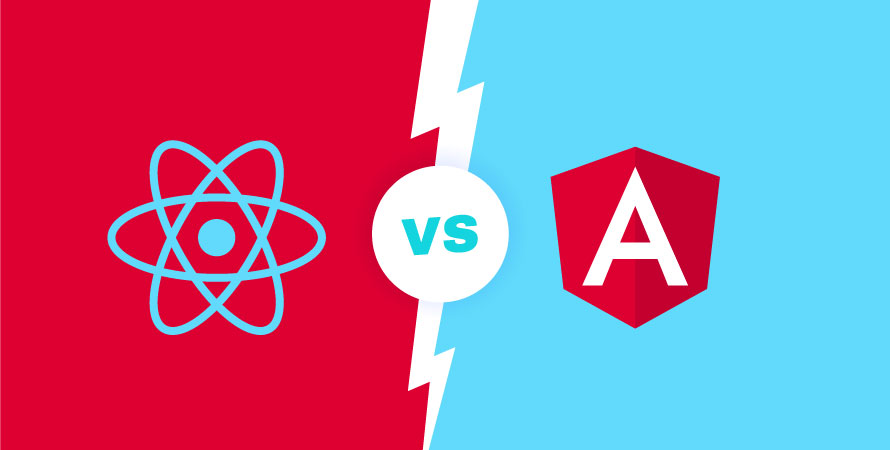
When it comes to front-end development, ReactJS and AngularJS stand out as two of the most popular frameworks. Developed by Facebook, React Javascript is a JavaScript library designed for building user interfaces with a focus on the efficient rendering of components. AngularJS, on the other hand, is a comprehensive framework created by Google that facilitates the development of web applications using TypeScript.
Choosing the right framework is crucial for developers and businesses alike. The choice can significantly impact the application’s performance, scalability, and maintainability.
Key criteria for comparing ReactJS and AngularJS include:
- Architecture: Understanding the core architecture of each framework.
- Data Binding: How each framework handles data binding.
- Rendering Approach: The methodologies used for rendering updates.
- Testing & Debugging: Tools and techniques available for maintaining code quality.
- Performance: How well each framework performs under various conditions.
- Community & Ecosystem: Availability of resources, support, and third-party libraries.
- Scalability: Capabilities to handle large-scale applications.
Here, in this blog, you will learn about different angular versions and what is react framework.
ReactJS
ReactJS, developed by Facebook, is a popular JavaScript library for building user interfaces. Its architecture is highly regarded for its flexibility and efficiency.
Component-Based Architecture
ReactJS uses a component-based architecture. This means that the UI is broken down into reusable components, each managing its state and lifecycle. Components can be nested, managed, and handled independently, which fosters modularity and makes it easier to maintain complex applications.
Virtual DOM
A significant feature in ReactJS is the use of the virtual DOM. Unlike the real DOM, which directly interacts with the browser’s rendering engine, the virtual DOM is a lightweight copy maintained in memory.
- When a component’s state changes, ReactJS updates the virtual DOM first.
- It then compares this updated virtual DOM with a snapshot of the previous state (a process known as diffing).
- Only the differences between these two states are applied to the real DOM.
This approach results in efficient updates and minimizes expensive direct manipulations of the real DOM.
Declarative Programming
ReactJS embraces declarative programming, where developers describe what they want rather than how to achieve it. This approach contrasts with imperative programming used in many other frameworks.
- Developers define the desired state of the UI, and ReactJS handles rendering to match this state.
- This leads to more predictable code and easier debugging.
Advantages:
- Simplicity: Declarative code tends to be more readable and easier to understand.
- Predictability: By focusing on what the UI should look like at any given point in time, developers can avoid many classes of bugs related to state management.
- Debugging: Since the flow of data is more predictable, debugging becomes more straightforward.
Data Binding
Data binding is a fundamental concept in ReactJS that plays a crucial role in how data moves within an application. Unlike some other frameworks, ReactJS uses a one-way data binding approach, which has significant implications for how components interact and update.
One-Way Data Binding
In ReactJS, data flows in a one-way direction from parent components to child components. Here’s how it works:
- Parent Components: Hold the state and pass it down as props to child components.
- Child Components: Receive the state as props and can render UI based on these props but cannot directly modify the state of their parent component.
javascript class ParentComponent extends React.Component { constructor() { super(); this.state = { message: “Hello” }; } render() { return ; } }
const ChildComponent = (props) => { return {props.message}; };
This one-way data flow ensures that the source of truth for the data is clear and predictable, making it easier to debug issues and creating more robust applications.
Benefits of One-Way Data Binding
The use of one-way data binding in ReactJS offers several advantages:
- Predictability: Since data can only flow in one direction, it’s easier to track the flow of information through the application.
- Performance: Simplifies state management, reducing unnecessary re-renders and improving performance.
- Maintainability: Code is easier to maintain due to its structured and predictable nature.
Two-Way Data Binding in ReactJS
While ReactJS doesn’t have built-in support for two-way data binding, developers can achieve a similar effect by using controlled components. In this pattern, input elements are controlled by React state, allowing for both reading and updating of form values.
javascript class ControlledComponent extends React.Component { constructor() { super(); this.state = { inputValue: “” }; }
handleChange = (event) => { this.setState({ inputValue: event.target.value }); };
render() { return ( ); } }
With controlled components, any changes made by the user in the input field will update the state, and vice versa. This approach combines the benefits of one-way data binding with the flexibility of two-way interaction for specific use cases involving forms or user inputs.
Rendering Approach
Javascript React is an open-source javascript framework, a JavaScript library developed by Facebook that revolutionizes rendering through its use of the virtual DOM. This approach is central to its efficiency and performance in building user interfaces.
Virtual DOM in ReactJS:
The virtual DOM is a lightweight, in-memory representation of the real DOM. Here’s how it works:
- When the state of a component changes, Facebook React creates a new virtual DOM tree.
- It then compares this new virtual DOM with the previous one using a process called “reconciliation.”
Steps in ReactJS Rendering Process:
Here are the key steps involved in rendering with ReactJS:
- State Change: A change in data triggers the creation of a new virtual DOM tree.
- Diffing Algorithm: ReactJS compares the new virtual DOM with the old one to find differences.
- Patch Application: Only the differences (or patches) are updated on the real DOM. This selective update process is what makes React’s rendering highly efficient.
AngularJS
Angular framework, a powerful JavaScript framework developed by Google, is widely used for web app development. Its architecture is built around the concept of components, which are the basic building blocks of an AngularJS application.
Component-Based Architecture in AngularJS
AngularJS uses a component-based architecture where each component encapsulates the following:
- Template: Defines the view and layout.
- Controller: Handles the business logic.
- Service: Manages data and reusable logic.
This architecture promotes modularity, making it easier to manage and scale applications by breaking them down into smaller, reusable pieces. For example, an e-commerce application can have separate components for the product list, shopping cart, and checkout process.
Key Features of AngularJS Architecture
- Dependency Injection: AngularJS uses dependency injection to manage components’ dependencies, improving code maintainability and testability. Dependencies are declared and injected at runtime rather than being hard-coded.
- Two-Way Data Binding: The bidirectional data flow between the model and view ensures that any changes in the model are instantly reflected in the view and vice versa. This feature simplifies synchronization between the user interface and data model.
- Directives: Directives extend HTML’s capabilities by allowing developers to create custom elements, attributes, and behaviors. Commonly used directives include ngIf, ngFor, and ngClass.
- Services: These singletons provide shared functionality across components. Services can be used for tasks such as fetching data from APIs or sharing state between different parts of an application.
- Modules: AngularJS applications are organized into modules. Each module encapsulates related components, services, filters, directives, and other elements. This modular approach facilitates code organization and reuse.
Comparison with ReactJS Architecture
While both AngularJS and ReactJS utilize component-based architectures, there are several differences:
- Data Binding: AngularJS uses two-way data binding which automatically synchronizes data between the model and view. In contrast, ReactJS uses one-way data binding (unidirectional flow), providing better control over data but requiring more boilerplate code for updates.
- Templates: AngularJS employs declarative templates using HTML enhanced with directives. ReactJS utilizes JSX, a syntax that combines JavaScript with HTML-like tags.
- Dependency Injection: This core feature in AngularJS is absent in ReactJS. Instead, React relies on context APIs or third-party libraries for dependency management.
Data Binding
Data binding in Angular JS is one of its core features, setting it apart from other JavaScript frameworks. Developed by Google, AngularJS vs angular uses a powerful mechanism known as two-way data binding. This feature ensures synchronization between the model and the view, meaning any changes in the user interface are instantly reflected in the underlying data model and vice versa.
How Two-Way Data Binding Works?
- Model to View: When the data in the model changes, AngularJS updates the view automatically. For instance, if a variable representing user input is updated, the corresponding element in the UI reflects this change without additional code.
- View to Model: Conversely, when a user interacts with the view (e.g., entering text in an input field), AngularJS updates the model directly. This eliminates the need for manual DOM manipulation or event handling.
Benefits of Two-Way Data Binding
- Reduced Boilerplate Code: The automatic synchronization minimizes repetitive coding tasks, making development faster and more efficient.
- Enhanced Readability: Code becomes more readable and maintainable since developers don’t need to write extensive code for DOM manipulation.
- Real-time Updates: Ensures that changes are immediately visible in both directions, providing a seamless user experience.
Conclusion
Choosing between ReactJS and AngularJS depends on factors like project complexity, learning curve, performance, and community support. ReactJS is ideal for dynamic, content-heavy applications, offering simplicity and a component-based approach, making it easier to learn and efficient in rendering with its virtual DOM.
AngularJS, better suited for large-scale projects, has a comprehensive framework but a steeper learning curve and can be slower due to real DOM manipulation in complex apps. Both frameworks have robust community support, with ReactJS often having a larger ecosystem. Evaluating these aspects will help you decide which framework is best for your future projects.