Mastering State Management in React.js Framework
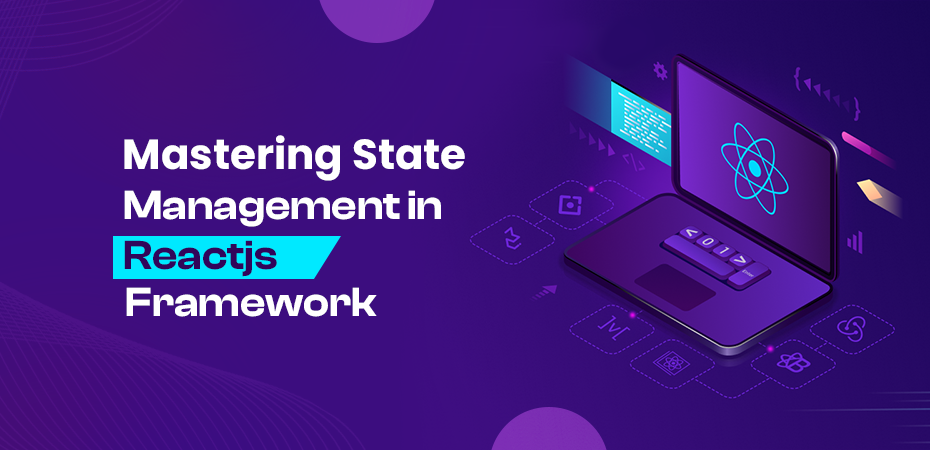
State management in React.js is a critical aspect of building dynamic and responsive applications. React state management involves organizing and controlling the dynamic data (state) within a React application. Without proper state management, applications can quickly become unmanageable, leading to bugs and performance issues.
Mastering ReactJS state management is essential for building scalable applications. As your application grows, so does the complexity of the state it needs to manage. Efficient state management ensures that your application remains maintainable and performant, even as it scales.
In this comprehensive React.Js State Management Guide, you will learn:
- The fundamentals of state in React and its importance.
- How to manage local component state effectively using the useState hook.
- Various approaches to global state management, including Context API and third-party libraries like Redux, MobX, and Recoil.
- Advanced techniques such as the reducer pattern with useReducer and modeling complex states with XState..
- Performance optimization strategies to reduce unnecessary re-renders and improve efficiency.
This guide will provide you with the necessary tools and knowledge.
Understanding State in React
State is a fundamental concept in React that plays a crucial role in building dynamic and interactive applications. In essence, state refers to the data that determines the behavior and rendering of components. By managing the state of JS effectively, developers can create applications that respond to user input, update in real-time, and maintain consistency across different views.
Definition and Role of State in Building Dynamic Applications
In React, using state represents the mutable aspects of components. Unlike props, which are immutable and passed from parent to child components, state is managed within the component itself. By utilizing control state, components can:
- Store user inputs: For example, form fields can store user inputs temporarily before submission.
- Track component visibility: Toggle elements like modals or dropdowns based on user actions.
- Maintain application status: Keep track of whether data is being loaded, or if an operation was successful.
The primary way to manage state within a component is through the useState hook:
jsx import React, { useState } from ‘react’;
function ExampleComponent() { const [count, setCount] = useState(0);
return ( You clicked {count} times <button onClick={() => setCount(count + 1)}>Click me ); }
Difference Between Local and Global State
Understanding the distinction between local and global state is vital for effective state management:
Local State: Managed within individual components using hooks like useState. It is ideal for scenarios where state does not need to be shared across multiple components. For instance:
- Form inputs
- Component-specific UI states (e.g., toggled visibility)
Global State: Managed at a higher level and shared across multiple components. This is necessary when multiple parts of the application need access to or need to modify the same piece of data. Examples include:
- User authentication status
- Theme settings (light/dark mode)
React provides several tools for managing global state, such as Context API and third-party libraries like Redux.
By understanding what is state in React and its various forms, developers can better architect their applications to be scalable and maintainable.
Local State Management with useState Hook
Managing Local Component State with useState Hook
The useState hook is the cornerstone of managing local component state in React. It allows you to add state to functional components, which was previously only possible in class components. By calling the useState function, you can create a piece of state that React will preserve between re-renders. You will learn how to set setactiveindex in React utilizing this useState Hook.
javascript import React, { useState } from ‘react’;
function Counter() { const [count, setCount] = useState(0);
return ( You clicked {count} times <button onClick={() => setCount(count + 1)}>Click me ); }
In the example above:
- The useState hook initializes the count state variable with a value of 0.
- The setCount function is used to update the value of count.
Best Practices for Effective Local State Management
Effective management of local state involves several best practices:
Keep State Minimal: Store only the information necessary for rendering. Derived data should be computed as needed.
Use Multiple State Variables: Instead of one complex state object, use state React simpler state variables.
simpler state variables.
javascript const [firstName, setFirstName] = useState(”); const [lastName, setLastName] = useState(”);
Avoid Deeply Nested State: Deeply nested objects can make updates cumbersome. Flatten your state structure when possible.
javascript // Instead of this const [formData, setFormData] = useState({ name: { first: ”, last: ” } });
// Consider this const [firstName, setFirstName] = useState(”); const [lastName, setLastName] = useState(”);
Memoize Callbacks: Use callbacks and memoization techniques like useCallback and useMemo to prevent unnecessary re-renders.
Use Cases Where Local State is Sufficient
Local state managed by the useState hook is ideal for scenarios where:
- Isolated Component Logic: The state is specific to an individual component and does not need to be shared across multiple components.
- Simple Forms: Handling form inputs within a single component without needing global state management.
- Component-Specific UI States: Managing UI states like modals visibility or tab selections within a specific component. You will know how to reset level capital.
React JS Developers can maintain cleaner and more efficient React applications by adhering to these best practices and understanding the appropriate use cases for the local state.
Global State Management Approaches in React
1. Context API for Managing Shared State
The Context API, a built-in feature of React, provides an efficient way to manage shared state across multiple components without prop drilling. Prop drilling involves passing state through several layers of components, which can become cumbersome and lead to unmanageable code as an application grows. Context API addresses this by allowing you to create a global state that any component within the context can access directly.
Benefits of Using Context API:
- Simplified State Sharing: By eliminating the need for prop drilling, Context API makes it easier to share state across deeply nested components.
- Enhanced Readability: With the state being centralized in context providers, code readability and maintainability are significantly improved.
- Flexibility: The ability to update state from any component within the provider’s scope offers great flexibility in managing application data flow.
Limitations of Context API:
- Performance Concerns: Since every component subscribed to the context re-renders when the context value changes, using Context API for large-scale applications can lead to performance issues.
- Single Responsibility Principle: Overusing Context API might lead to components handling more than one responsibility, violating the single responsibility principle.
Implementing Context API Effectively:
To demonstrate how to implement Context API effectively, let’s walk through a simple example where we create a global theme context.
Create the Context:
javascript import React, { createContext, useState } from ‘react’;
export const ThemeContext = createContext();
const ThemeProvider = ({ children }) => { const [theme, setTheme] = useState(‘light’);
return ( <ThemeContext.Provider value={{ theme, setTheme }}> {children} </ThemeContext.Provider> ); };
export default ThemeProvider;
Use the Context in Components:
javascript import React, { useContext } from ‘react’; import { ThemeContext } from ‘./ThemeProvider’;
const ThemeSwitcher = () => { const { theme, setTheme } = useContext(ThemeContext);
return ( <button onClick={() => setTheme(theme === ‘light’ ? ‘dark’ : ‘light’)}> Switch to {theme === ‘light’ ? ‘dark’ : ‘light’} mode ); };
const App = () => ( <div className={app ${theme}}> Current Theme: {theme} );
In this example:
- We define a ThemeContext using createContext and wrap our application with a ThemeProvider component.
- The ThemeProvider holds the state (theme) and the function (setTheme) to update it.
- Any component within ThemeProvider can access and modify the theme by subscribing to ThemeContext.
This approach ensures that our theme state is globally available and manageable without prop drilling.
2. Redux: A Powerful State Management Library
Redux is a popular library for managing state in complex React applications. It is widely used because it can handle intricate state management needs in a structured and predictable way.
Centralized Store Concept in Redux
At the core of Redux is the centralized store. This store contains the entire state of the application, making it accessible to any component that needs it. This concept brings several advantages:
- Consistency: Having a single source of truth ensures that all components reflect the same state, reducing inconsistencies.
- Debugging: Centralizing state makes debugging easier as you can track how the state changes over time using tools like Redux DevTools.
- Scalability: As applications grow, maintaining and understanding state transitions becomes simpler with a centralized approach.
Pros and Cons of Adopting Redux in Your Projects
While Redux offers many benefits, it’s essential to weigh its pros and cons to determine if it’s the right fit for your project.
Pros:
- Predictable State Management: Redux enforces a predictable pattern by using actions and reducers, which helps maintain consistent behavior across your application.
- Middleware Integration: Middleware like redux-thunk or redux-saga enhances Redux’s capabilities by handling asynchronous actions effectively.
- Community and Ecosystem: A vast ecosystem of tools, libraries, and community support makes working with Redux more manageable.
Cons:
- Boilerplate Code: One of the primary criticisms of Redux is the amount of boilerplate code required to set up actions, reducers, and the store.
- Learning Curve: For newcomers, understanding concepts like reducers, actions, and middlewares can be challenging.
- Overkill for Small Projects: For smaller applications or those with simple state management needs, using Redux might add unnecessary complexity.
When choosing between global and local state management techniques like Context API or Redux, consider factors such as the complexity of your application’s state, team familiarity with these tools, and project scalability requirements. Both Context API and Redux have their unique benefits and use cases. Understanding these will help you make an informed decision on which approach best fits your project’s needs.
3. Exploring Other Third-Party Libraries for State Management
React’s ecosystem offers several third-party libraries that provide alternative approaches to managing complex states. These React state management libraries offer unique features and benefits that cater to different use cases and performance needs.
MobX
MobX is a reactive state management library that uses observables to track state changes. It allows components to automatically re-render when the observed state changes, providing a seamless reactive programming model.
Key Features:
- Observable State: Automatically tracks changes in state and updates components.
- Computed Values: Derive values from the state that update automatically when dependencies change.
- Actions: Encapsulate state modifications in functions for better maintainability.
Use Cases:
- Applications requiring highly dynamic UIs with frequent state updates.
- Projects where simplicity and minimal boilerplate code are priorities.
Recoil
Recoil introduces a novel approach to global state management by treating state as atoms and selectors. Atoms represent pieces of state, while selectors derive computed states based on atoms or other selectors.
Key Features:
- Atoms: Smallest units of state, allowing fine-grained control over re-renders.
- Selectors: Functions that compute derived state, promoting efficient data flow.
- Concurrent Mode Compatibility: Designed to work seamlessly with React’s concurrent features.
Use Cases:
- Applications needing granular control over component re-renders.
- Scenarios where derived states and dependencies play a significant role.
Jotai
Jotai is a minimalist state-driven management library focusing on atomic states. It leverages React hooks to manage local and global states with straightforward APIs, making it easy to integrate into existing projects.
Key Features:
- Atomic State Management: Breaks down complicated lift interfaces into small, manageable units.
- Simple Integration: Easy to use with minimal boilerplate code.
- Composable State Logic: Combines different atoms for complex state logic.
Use Cases:
- Projects requiring lightweight and flexible state management solutions.
- Applications aiming for easy-to-maintain and scalable state management architecture.
Zustand
Zustand, meaning “state” in German, is another minimalist library focusing on simplicity and performance. It uses hooks to manage global states without the need for boilerplate or context providers.
Key Features:
- Simplicity: Minimal API surface for ease of use.
- Performance Focused: Optimized for fast updates and minimal re-renders.
- Modular Design: Allows for the store to be split into multiple parts for better organization.
Use Cases:
- Lightweight applications needing efficient global state management.
- Projects where reducing complexity and improving performance are crucial.
Advanced State Management Techniques in React
The Reducer Pattern for Handling Complex States with useReducer Hook
Managing state becomes increasingly intricate as applications grow. The reducer pattern offers a robust solution for handling more complex states, especially when using the useReducer hook.
How the Reducer Pattern Works?
The reducer pattern is based on the concept of a reducer function, which takes two parameters:
- Current state
- Action
The reducer function processes these inputs and returns a new state. This pattern is useful for managing multiple related pieces of state or when state transitions are driven by specific actions.
javascript import React, { useReducer } from ‘react’;
const initialState = { count: 0 };
function reducer(state, action) { switch (action.type) { case ‘increment’: return { count: state.count + 1 }; case ‘decrement’: return { count: state.count – 1 }; default: throw new Error(); } }
function Counter() { const [state, dispatch] = useReducer(reducer, initialState);
return ( <> <button onClick={() => dispatch({ type: ‘decrement’ })}>- {state.count} <button onClick={() => dispatch({ type: ‘increment’ })}>+ </> ); }
In this example, the Counter component uses the useReducer hook to manage its state. Actions dispatched to the reducer function (increment and decrement) determine how the state should change.
When to Choose the Reducer Pattern?
Consider using the reducer pattern and useReducer hook in scenarios where:
- State logic is complex and dependent on multiple actions.
- Multiple components need to interact with shared state.
- You prefer a more predictable and maintainable way of managing states compared to useState.
Modeling Complex States with XState Library
When dealing with even more intricate states, finite state machines (FSMs) provided by libraries like XState can be invaluable.
Introduction to XState and Finite State Machines
XState allows developers to model application states as FSMs. This approach ensures that each possible state and transition between states is explicitly defined, reducing ambiguity and enhancing predictability.
javascript import { Machine } from ‘xstate’;
const toggleMachine = Machine({ id: ‘toggle’, initial: ‘inactive’, states: { inactive: { on: { TOGGLE: ‘active’ } }, active: { on: { TOGGLE: ‘inactive’ } } } });
In this example, a simple toggle machine is defined with two states (inactive and active) and transitions triggered by a TOGGLE event.
Benefits of Using Finite State Machines
Finite state machines offer several advantages:
- Predictability: Each state transition is clearly defined, preventing unexpected behaviors.
- Debuggability: Easier to debug as you can trace every state transition.
- Clarity: Improved clarity in how an application can move between different states.
Implementing XState in React
To integrate XState into a React application:
- Install XState:
- bash npm install xstate
- Use the defined machine within your component:
- javascript import React from ‘react’; import { useMachine } from ‘@xstate/react’; import { Machine } from ‘xstate’;
- const toggleMachine = Machine({ id: ‘toggle’, initial: ‘inactive’, states: { inactive: { on: { TOGGLE: ‘active’ } }, active: { on: { TOGGLE: ‘inactive’ } } } });
- function Toggle() { const [current, send] = useMachine(toggleMachine);
- return ( <button onClick={() => send(‘TOGGLE’)}> {current.matches(‘inactive’) ? ‘Off’ : ‘On’} ); }
- export default Toggle;
This setup ensures that your component adheres strictly to the defined states and transitions within the machine.
Incorporating advanced techniques like the reducer pattern and XState’s FSMs empowers developers to handle complex states effectively while maintaining clear and predictable behavior across their applications. These tools are essential for building scalable React applications that are easy to manage and debug.
Performance Optimization Strategies for State Management
Effective activestate management is crucial for maintaining performance in large-scale React applications. Employing performance optimization techniques for Reactjs setstate ensures a seamless user experience and efficient resource utilization.
Strategies for Optimizing Performance
1. Memoization
- Utilize the useMemo hook to memoize expensive calculations, ensuring they are only recalculated when necessary.
- Example: javascript const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
2. Selective Updates
- Leverage the shouldComponentUpdate lifecycle method in class components or React.memo for functional components to prevent unnecessary re-renders.
- Example: javascript const MemoizedComponent = React.memo(MyComponent);
3. Batching State Updates
Group multiple state updates into a single update to minimize rendering overhead.
Example: javascript setState((prevState) => ({ …prevState, key: newValue }));
4. Avoiding Inline Functions and Objects
Define functions and objects outside of render methods to prevent them from being recreated on each render.
Example: javascript const handleClick = () => { /* logic */ }; return Click Me;
Reducing Unnecessary Re-Renders
1. Using Keys Wisely
Ensure unique keys for list items to help React identify which items have changed.
Example: javascript {items.map(item => )}
2. Splitting Component Logic
- Break down large components into smaller, reusable components to isolate state and minimize re-rendering scope.
3. Optimizing Context Usage
- Avoid overusing Context API by localizing state as much as possible and only lifting state when absolutely necessary.
4. Throttling and Debouncing Events
- Implement throttling or debouncing techniques on high-frequency events like scroll or input events to reduce the number of state updates.
- Example using lodash: javascript const handleScroll = _.throttle(() => { /* logic */ }, 300);
Incorporating these strategies ensures that your React applications remain performant even as they scale in complexity and size.
Conclusion
Having explored various next JS state management strategies, it’s time to delve deeper into advanced concepts. Consider investigating server-side rendering support or tailored testing strategies for the approaches discussed.
Enhance your skills by learning how state management React integrates within the Next.js framework. Look for in-depth resources or courses on topics like Redux middleware, MobX observables, or XState transitions. Mastering these areas will solidify your understanding and application of state management, preparing you for building robust, scalable applications.