How to Develop a Video Game App Using React JS in 2024
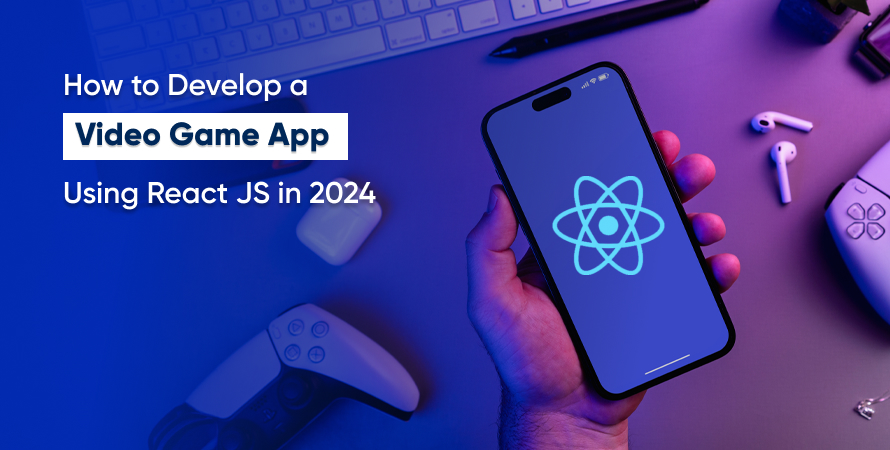
Introduction
The world of video game app development is always changing. With more and more gamers around the world, it’s incredibly important to create video game apps that are both fun and well-made. As we enter 2024, the tools and technologies available for making these apps are getting better.
That’s where React JS comes in. This JavaScript library is great for building user interfaces, but it can also be used to make awesome video game apps. React JS’s component-based structure and efficient rendering abilities make it a good choice for game developers who want to create interactive games.
In this article, we’ll look at how you can make a video game app using React JS in 2024. We’ll cover everything you need to know, including:
- Why React JS is important for making games.
- How to plan and design your project thoroughly.
- The process of coding, testing, and deploying your app.
- Step-by-step instructions for creating popular games like Tic Tac Toe and Candy Crush.
- Adding advanced features like high scores and multiplayer options to your games.
By the end of this guide, you’ll have all the information you need to start making your own video game apps with React JS. Let’s get started!
Understanding React JS for Game Development
React JS is a powerful JavaScript library used for building user interfaces, particularly single-page applications. In game development, React JS offers a robust framework for creating interactive and dynamic gaming experiences.
Significance of React JS in Game Development
Using React JS in game development allows developers to leverage its component-based architecture. This structure enables the creation of reusable components that can be efficiently managed and updated. For instance, in a game like Tic Tac Toe, each cell on the board can be a separate component, making the development process modular and manageable.
Advantages of Using React JS for Developing Video Game Apps
- Component Reusability: Components can be reused across different parts of the application, reducing redundancy.
- Virtual DOM: Enhances performance by updating only the parts of the DOM that have changed.
- State Management: Tools like useState and useReducer simplify state management, crucial for handling game logic.
- Developer Tools: React’s ecosystem includes powerful tools like Redux for state management and Jest for testing, which streamline development workflows.
Overview of JavaScript’s Role in React JS Development
JavaScript is the backbone of React JS, providing the language’s foundational syntax and features. Understanding JavaScript is essential for utilizing React effectively:
- ES6 Features: Familiarity with ES6 (e.g., arrow functions, destructuring) is crucial as they are extensively used in React.
- Asynchronous Programming: Techniques such as async/await and Promises are vital for handling API calls and asynchronous operations within games.
- Event Handling: Mastery over JavaScript event handling is necessary to manage user interactions within the game.
Leveraging these advantages and understanding JavaScript’s role enhances your capability to develop sophisticated video game apps using React JS.
Project Planning, Design, and Selecting the Right Tech Stack
Importance of Comprehensive Project Planning in Game Development with React JS
Effective project planning is essential for the success of any video game app development project. With React JS, detailed planning ensures that you can fully leverage the framework’s capabilities. Proper planning helps in identifying potential challenges early and mapping out a clear roadmap to navigate through them.
Steps Involved in Project Planning for a Video Game App using React JS
- Define Objectives: Establish clear goals for your game app.
- Research and Analysis: Analyze market trends, target audience preferences, and competitor apps.
- Scope Documentation: Document the features, functionalities, and limitations of your game.
- Resource Allocation: Determine required resources including team members, tools, and timeframes.
- Milestone Setting: Break down the project into manageable phases with specific deliverables.
Overview of Wireframing and Design Process for Creating Engaging Game Interfaces
Creating engaging game interfaces requires meticulous wireframing and design:
- Wireframing: Sketch out the user interface layout to visualize game screens and user interactions.
- Design Mockups: Develop high-fidelity designs to showcase the look and feel of your game.
- User Experience (UX): Focus on intuitive navigation and immersive experiences.
- Prototyping: Create interactive prototypes to test user flows before actual development.
Tips for Selecting the Right Tech Stack and Utilizing Version Control in Collaborative Game Development
Choosing the right tech stack is crucial:
- Front-End Framework: React JS for its reusability and component-based architecture.
- Back-End Solutions: Node.js or Firebase for real-time data processing.
- APIs: Integrate APIs like RAWG Video Games API for dynamic content.
Implementing version control is equally important:
“Version control systems like Git enable collaborative coding by tracking changes, allowing multiple developers to work simultaneously without conflicts.”
Use platforms such as GitHub or Bitbucket to manage code repositories effectively, ensuring seamless collaboration among team members.
This structured approach will streamline your development process, making it easier to build a robust video game app with React JS.
Coding, Testing, and Deployment
Coding Process for a Video Game App using React JS
When developing a video game app with React JS, the coding phase is critical. You begin by setting up your development environment with tools like Node.js, npm, and Create React App. These tools streamline the initialization process.
1. Initialize the Project
bash npx create-react-app my-game-app cd my-game-app
2. Component Structure
Break down your game into reusable components. For instance, in a Tic Tac Toe game, you might have components like Board, Square, and Game.
3. State Management
Utilize React’s state management capabilities with hooks like useState and useEffect to manage game states and lifecycle events.
Best Practices in Coding
- Modular Code: Keep your code modular by breaking down large components into smaller, manageable ones.
- Reusable Components: Create reusable components to avoid redundancy.
- Consistent Naming Conventions: Use consistent naming conventions for variables and functions.
Example of a simple component structure:
jsx import React, { useState } from ‘react’;
function Square({ value, onClick }) { return ( {value} ); }
function Board() { const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick(i) { const newSquares = squares.slice(); newSquares[i] = ‘X’; // Example logic for player move setSquares(newSquares); }
return ( {squares.map((square, i) => ( <Square key={i} value={square} onClick={() => handleClick(i)} /> ))} ); }
Testing
Testing ensures that your game functions as expected. Utilize libraries like Jest and React Testing Library to write unit tests for your components.
- Unit Tests: Focus on individual units of code.
- Integration Tests: Ensure different parts of the application work together.
- End-to-End Tests: Simulate real user interactions to verify the entire application flow.
Example of a simple test using Jest:
jsx import { render, fireEvent } from ‘@testing-library/react’; import Board from ‘./Board’;
test(‘renders Board component’, () => { const { getByText } = render(); fireEvent.click(getByText(/X/i)); // Simulate click event });
Deployment
Deploying your React JS game app involves several steps:
1. Build the App
bash npm run build
2. Choose a Hosting Service
Platforms like Netlify, Vercel, or GitHub Pages offer easy deployment options.
3. Deploy Command for Netlify
bash netlify deploy –prod
By following these steps, you ensure a smooth transition from development to deployment, making your game accessible to users worldwide.
Building a Tic Tac Toe Game with React Native
Creating a Tic Tac Toe game using React Native involves several steps. This guide will walk you through the process, providing code snippets and explanations along the way.
Step 1: Setting Up Your Project
First, initiate a new React Native project:
bash npx react-native init TicTacToeGame cd TicTacToeGame
Step 2: Creating the Game Components
You’ll need components for the game board and individual squares. Start by creating a Square component:
javascript // Square.js import React from ‘react’; import { TouchableOpacity, Text, StyleSheet } from ‘react-native’;
const Square = ({ value, onPress }) => ( {value} );
const styles = StyleSheet.create({ square: { width: 100, height: 100, justifyContent: ‘center’, alignItems: ‘center’, borderWidth: 1, }, text: { fontSize: 24, }, });
export default Square;
Step 3: Building the Board Component
Next, create the Board component to manage the state and render Square components:
javascript // Board.js import React, { useState } from ‘react’; import { View, StyleSheet } from ‘react-native’; import Square from ‘./Square’;
const Board = () => { const [squares, setSquares] = useState(Array(9).fill(null)); const [xIsNext, setXIsNext] = useState(true);
const handlePress = (i) => { if (squares[i]) return; const newSquares = squares.slice(); newSquares[i] = xIsNext ? ‘X’ : ‘O’; setSquares(newSquares); setXIsNext(!xIsNext); };
return ( {squares.map((square, i) => ( <Square key={i} value={square} onPress={() => handlePress(i)} /> ))} ); };
const styles = StyleSheet.create({ board: { flexDirection: ‘row’, flexWrap: ‘wrap’, width: 300, }, });
export default Board;
Step 4: Rendering the Game
Finally, render the Board component in your main application file:
javascript // App.js import React from ‘react’; import { SafeAreaView } from ‘react-native’; import Board from ‘./Board’;
const App = () => ( );
export default App;
Building a Candy Crush Clone with React Native
Creating a Candy Crush Clone with React Native can be an exciting project that enhances your game development skills. Here’s a detailed guide to help you build this game from scratch.
Step-by-Step Guide
1. Initialize the Project
- Use npx react-native init CandyCrushClone to set up a new React Native project.
- Install necessary dependencies: npm install react-native-gesture-handler react-native-reanimated.
2. Set Up the Game Board
- Create a grid layout for the candies using Flexbox.
- Define state variables to manage the candies and their positions using useState hooks.
javascript const [candies, setCandies] = useState(initialCandies); const [board, setBoard] = useState(generateInitialBoard());
3. Implement Candy Movement
- Use touch gestures to enable candy swapping.
- Utilize react-native-gesture-handler to track user touch events.
javascript const handleTouch = (index) => { // Code to handle candy swap };
4. Check for Matches
- Write logic to detect matches of three or more candies in a row or column.
- Update the state to reflect these changes and drop new candies into place.
javascript const checkForMatches = () => { // Code to check and remove matched candies };
5. Add Animations
- Incorporate animations for candy movements using react-native-reanimated.
- Enhance user experience by making the game visually appealing.
6. Scoring System
- Implement a scoring system that updates as the player makes successful matches.
javascript const [score, setScore] = useState(0);
const updateScore = (points) => { setScore(score + points); };
7. Testing and Debugging
- Test the game thoroughly on different devices.
- Debug any issues that arise during gameplay.
8. Deploying the Game
- Once satisfied with the functionality and performance, prepare your app for deployment.
- Use expo eject if you started with Expo, or follow standard React Native deployment procedures.
Building a Candy Crush Clone with React Native not only hones your coding skills but also introduces you to essential concepts in game development like state management, animations, and user interactions.
Implementing High Scores, Multiplayer Functionality, and Publishing the Game
High Score Functionality
Incorporating high score functionality in a video game app creates a competitive edge. Using React Native, you can manage high scores with state management and persistent storage.
State Management
javascript const [highScores, setHighScores] = useState([]);
Persistent Storage
Utilize AsyncStorage to store and retrieve high scores.
javascript import AsyncStorage from ‘@react-native-async-storage/async-storage’;
const storeHighScore = async (score) => { try { await AsyncStorage.setItem(‘HIGH_SCORE’, JSON.stringify(score)); } catch (error) { console.error(‘Error storing high score’, error); } };
const getHighScore = async () => { try { const score = await AsyncStorage.getItem(‘HIGH_SCORE’); return score ? JSON.parse(score) : []; } catch (error) { console.error(‘Error retrieving high score’, error); } };
Building Multiplayer Games with React Native
Creating multiplayer functionality enhances user engagement. React Native integrates well with services like Firebase to manage real-time data.
Firebase Setup
bash npm install –save @react-native-firebase/app @react-native-firebase/firestore
Multiplayer Logic
javascript import firestore from ‘@react-native-firebase/firestore’;
const createRoom = async () => { const roomRef = await firestore().collection(‘rooms’).add({ players: 1, // other game settings }); return roomRef.id; };
const joinRoom = async (roomId) => { const roomRef = firestore().collection(‘rooms’).doc(roomId); await roomRef.update({ players: firestore.FieldValue.increment(1), }); };
Publishing the Game
Publishing your game on popular app stores such as the App Store requires several steps:
Prepare Your App: Ensure all assets, descriptions, and screenshots are ready.
Build for Production:
- iOS: npx react-native run-ios –configuration Release
- Android: npx react-native run-android –variant=release
Submit to App Stores:
App Store:
- Register an Apple Developer account.
- Use Xcode to archive and upload your app.
- Complete app information in App Store Connect.
Google Play Store:
- Register a Google Play Developer account.
- Use Android Studio to generate the APK/AAB file.
- Upload and complete the app information on the Google Play Console.
Incorporating these elements will elevate your game development project using React Native, ensuring competitive features and accessibility on major platforms.
Conclusion
Starting your journey in game development with React JS in 2024 offers immense opportunities. The tools and techniques discussed in this tutorial empower you to create engaging and interactive experiences. Embrace the knowledge shared here, and take bold steps towards building your own video game apps. The world of gaming is vast and evolving, and React JS stands as a robust platform to bring your creative visions to life. Dive into learning about game development with React JS in 2024 and start crafting your unique gaming experience today.