How to add Chatbot in React JS Project?
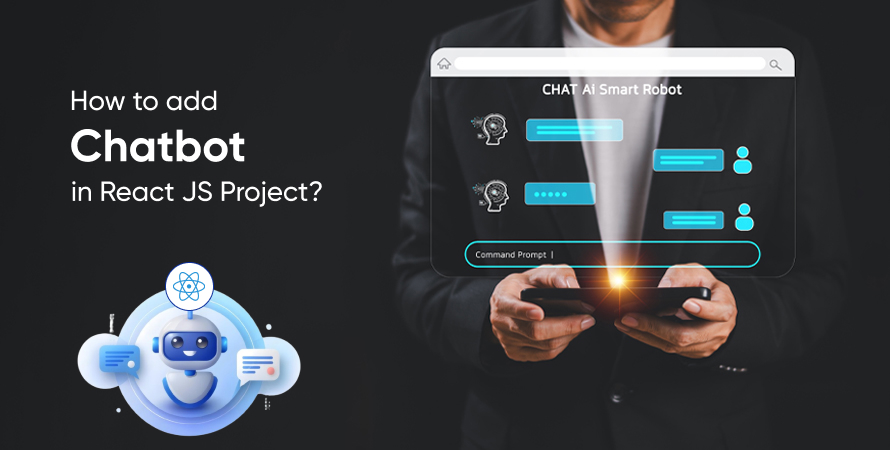
Chatbots have changed the way users interact with web applications. They provide instant responses and make communication seamless, improving the overall user experience. Chatbots can be used for various purposes, such as answering common questions or helping users with complex tasks.
Adding a chatbot to a React JS project can greatly enhance user engagement. React JS is known for its component-based structure, which makes it relatively easy to integrate a chatbot. Here are some key benefits of including a chatbot in your React JS project:
- 24/7 Availability: Chatbots are available to assist users at any time, not limited by business hours.
- Scalability: They can handle multiple conversations simultaneously without affecting performance.
- User Engagement: Chatbots conversationally interact with users, encouraging them to stay longer on your application.
- Efficiency: By automating repetitive queries, chatbots reduce the workload on customer support teams.
With these advantages, developers can create dynamic and responsive web applications that meet the needs of modern users. In this guide, we will show you how to create a chatbot for your React JS project using the react-simple-chatbot library.
Prerequisites
Before you can learn how to make a chatbot for your React JS project, there are a few things you need to do first to make sure everything goes smoothly.
Step 1: Create a New React JS Project
To begin, you’ll need to create a new React application using the Create React App. This handy tool gives you an easy way to develop and build your app.
bash npx create-react-app chatbot-project cd chatbot-project
This command sets up a brand new React project with the name chatbot-project and takes you into the project folder.
Step 2: Install the Necessary Modules
Next, you’ll need to install some modules that are essential for integrating the chatbot tutorial. We’ll be using a library called react-simple-chatbot, which is great because it’s simple to use and works well with React-chatbot-kit.
To install react-simple-chatbot, run this command:
bash npm install react-simple-chatbot –save
We’ll also need another module called styled-components to help us customize the appearance of the chatbot:
bash npm install styled-components –save
Once these modules are installed, your project will be all set for the next steps of adding and configuring the chatbot.
Step 1: Adding and Configuring react-simple-chatbot
React-simple-chatbot is a straightforward and highly customizable chatbot library for React applications. It provides an easy-to-use interface to integrate a React JS chatbot into your project, making it an excellent choice for developers at all levels. Its simplicity, combined with the ability to customize both appearance and behavior, makes it stand out from other available options.
Step 1.1: Installing the react-simple-chatbot Dependency
First, install the react-simple-chatbot dependency in your React JS project using npm or yarn:
bash npm install react-simple-chatbot –save
Alternatively, if you prefer yarn:
bash yarn add react-simple-chatbot
Step 1.2: Importing and Configuring the ThemeProvider
To customize the chatbot’s appearance, import ThemeProvider from styled-components. This allows you to define a theme object that customizes various aspects like background color, header background color, font size, and more.
Install styled-components if you haven’t already:
bash npm install styled-components –save
or
bash yarn add styled-components
Next, import ThemeProvider in your component file:
javascript import { ThemeProvider } from ‘styled-components’; import ChatBot from ‘react-simple-chatbot’;
Step 1.3: Defining the Theme Object
Define a theme object to customize the chatbot’s colors and font styles. Here’s an example:
javascript const theme = { background: ‘#f5f8fb’, fontFamily: ‘Helvetica Neue’, headerBgColor: ‘#00bfff’, headerFontColor: ‘#fff’, headerFontSize: ’15px’, botBubbleColor: ‘#00bfff’, botFontColor: ‘#fff’, userBubbleColor: ‘#fff’, userFontColor: ‘#4a4a4a’ };
Step 1.4: Configuring the Steps Array
The steps array defines the conversation flow of the ReactJS chatbot. Each step includes properties such as id, message, trigger, user input options, and possible outcomes.
Here’s an example configuration:
javascript const steps = [ { id: ‘1’, message: ‘Hello! What is your name?’, trigger: ‘2’ }, { id: ‘2’, user: true, trigger: ‘3’ }, { id: ‘3’, message: ‘Hi {previousValue}, nice to meet you!’, end: true } ];
Combining Everything
Now combine everything within your main component:
javascript import React from ‘react’; import ChatBot from ‘react-simple-chatbot’; import { ThemeProvider } from ‘styled-components’;
const App = () => ( );
export default App;
This setup provides a fully functional chatbot integrated into your React JS project with a customized appearance based on the defined theme object.
Step 2: Creating Project Structure for Chatbot
Importance of a Well-Structured Project Architecture
Designing a well-structured project architecture is crucial for maintaining and scaling your React JS application. A clear and organized structure makes it easier to manage components, debug issues, and add new features. This section will guide you through creating the necessary components and files to create your chatbot for free effectively within your React JS project.
Step 2.1: Creating Necessary Components and Files
To start, let’s create the essential components and files:
- Create a components directory: This directory will house all the reusable components. You will know how to build a chatbot.
- Create a Chatbot.js file within the components directory: This file will define the Chatbot create component.
Create additional files if needed, such as ChatbotSteps.js, to keep your steps array separate for better readability and maintenance.
Here’s an example of how your project structure might look:
plaintext my-react-app/ │ ├── src/ │ ├── components/ │ │ ├── Chatbot.js │ │ └── ChatbotSteps.js │ ├── App.js │ └── index.js ├── public/ └── package.json
Step 2.2: Defining the Steps Array
The steps array defines the conversation flow of your chatbot implementation. Each step in this array includes unique identifiers, messages, triggers, user input options, and possible outcomes.
Create a ChatbotSteps.js file to define the steps array:
javascript // src/components/ChatbotSteps.js
export const steps = [ { id: ‘1’, message: ‘Welcome to our site! How can I assist you today?’, trigger: ‘options’ }, { id: ‘options’, options: [ { value: ‘faq’, label: ‘FAQ’, trigger: ‘faq’ }, { value: ‘contact’, label: ‘Contact Us’, trigger: ‘contact’ } ] }, { id: ‘faq’, message: ‘Here are some frequently asked questions…’, end: true }, { id: ‘contact’, message: ‘You can reach us at contact@example.com.’, end: true } ];
Import the steps array into your Chatbot.js component:
javascript // src/components/Chatbot.js
import React from ‘react’; import ChatBot from ‘react-simple-chatbot’; import { ThemeProvider } from ‘styled-components’; import { steps } from ‘./ChatbotSteps’;
const theme = { background: ‘#f5f8fb’, headerBgColor: ‘#00bfff’, headerFontSize: ’20px’, botBubbleColor: ‘#00bfff’, botFontColor: ‘#fff’, userBubbleColor: ‘#fff’, userFontColor: ‘#4a4a4a’ };
const ChatbotComponent = () => ( );
export default ChatbotComponent;
By defining the steps array separately and importing it into your main chatbot component, you ensure that your code remains modular and easy to manage.
Step 3: Running the Application with Chatbot
Testing and validating the chatbot integration in your React JS application is crucial to ensure everything works as expected.
Step 3.1: Running the React JS Application with Integrated Chatbot
To start, you’ll need to run your React JS application. Open your terminal and navigate to the root directory of your project. Execute the following command:
bash npm start
This command will compile your project and launch it in your default web browser. You should see your application running with the chatbot interface integrated as per your configurations.
Step 3.2: Interacting with the Chatbot
Once the application is up and running, interact with the chatbot to assess its functionality. Here’s what you should do:
- Initiate a Conversation: Click on the chatbot icon or input area.
- Respond to Prompts: Follow through the conversation flow you’ve defined in the steps array.
- Provide Inputs: Test various user input scenarios to ensure all possible branches of conversation are covered.
Observe how each interaction triggers subsequent steps and how well the chatbot handles different user inputs.
Step 3.3: Verifying Desired Output
Ensure that all expected behaviors are functioning correctly:
- Message Flow: Confirm that messages appear in the correct sequence as defined.
- User Input Handling: Verify that user inputs are processed accurately.
- Customization: Check that visual customizations (colors, fonts) reflect your theme settings.
- Bot Responses: Ensure that bot responses align with defined triggers and outcomes.
If you notice any discrepancies or errors, revisit your steps array and configurations to make necessary adjustments. By thoroughly interacting with the chatbot and verifying its output, you can ensure a seamless integration within your React JS application.
Conclusion
Integrating a chatbot in your React JS project is just the beginning. Implementing NLP can help your chatbot understand and respond to user queries more naturally and effectively. Leveraging ML algorithms can enable your chatbot to learn from user interactions, improving its responses over time.
This guide to adding a chatbot in a React JS project is a foundational step towards creating richer, more interactive web applications. Embrace these advanced techniques and features to stay ahead in the dynamic world of web development.